COLLECTIONS
The Collection in Java is a framework that provides an architecture to store and manipulate the group of objects.
Hierarchy of Collection Framework
Iterator interface:
List Interface is the subinterface of Collection. It contains index-based methods to insert, access and delete elements. It is a factory of ListIterator interface.
List Interface declaration
Method | Description | |
---|---|---|
void add(int index, E element) | It is used to insert the specified element at the specified position in a list. | |
boolean add(E e) | It is used to append the specified element at the end of a list. | |
boolean addAll(Collection<? extends E> c) | It is used to append all of the elements in the specified collection to the end of a list. | |
boolean addAll(int index, Collection<? extends E> c) | It is used to append all the elements in the specified collection, starting at the specified position of the list. | |
void clear() | It is used to remove all of the elements from this list. | |
boolean equals(Object o) | It is used to compare the specified object with the elements of a list. | |
int hashcode() | It is used to return the hash code value for a list. | |
E get(int index) | It is used to fetch the element from the particular position of the list. | |
boolean isEmpty() | It returns true if the list is empty, otherwise false. | |
int lastIndexOf(Object o) | It is used to return the index in this list of the last occurrence of the specified element, or -1 if the list does not contain this element. | |
Object[] toArray() | It is used to return an array containing all of the elements in this list in the correct order. | |
It is used to return an array containing all of the elements in this list in the correct order. | ||
boolean contains(Object o) | It returns true if the list contains the specified element | |
boolean containsAll(Collection<?> c) | It returns true if the list contains all the specified element | |
int indexOf(Object o) | It is used to return the index in this list of the first occurrence of the specified element, or -1 if the List does not contain this element. | |
E remove(int index) | It is used to remove the element present at the specified position in the list. | |
boolean remove(Object o) | It is used to remove the first occurrence of the specified element. | |
boolean removeAll(Collection<?> c) | It is used to remove all the elements from the list. | |
void replaceAll(UnaryOperator | It is used to replace all the elements from the list with the specified element. | |
void retainAll(Collection<?> c) | It is used to retain all the elements in the list that are present in the specified collection. | |
E set(int index, E element) | It is used to replace the specified element in the list, present at the specified position. | |
void sort(Comparator<? super E> c) | It is used to sort the elements of the list on the basis of specified comparator. | |
Spliterator | It is used to create spliterator over the elements in a list. | |
List<E> subList(int fromIndex, int toIndex) | It is used to fetch all the elements lies within the given range. | |
int size() | It is used to return the number of elements present in the list. |
Java ListIterator Interface
ListIterator Interface declaration
Methods of Java ListIterator Interface:
Method | Description |
---|---|
void add(E e) | This method inserts the specified element into the list. |
boolean hasNext() | This method returns true if the list iterator has more elements while traversing the list in the forward direction. |
E next() | This method returns the next element in the list and advances the cursor position. |
int nextIndex() | This method returns the index of the element that would be returned by a subsequent call to next() |
boolean hasPrevious() | This method returns true if this list iterator has more elements while traversing the list in the reverse direction. |
E previous() | This method returns the previous element in the list and moves the cursor position backward. |
E previousIndex() | This method returns the index of the element that would be returned by a subsequent call to previous(). |
void remove() | This method removes the last element from the list that was returned by next() or previous() methods |
void set(E e) | This method replaces the last element returned by next() or previous() methods with the specified element. |
Java ArrayList class:![]() The important points about Java ArrayList class are:
Hierarchy of ArrayList class:
public class ArrayList<E> extends AbstractList<E> implements List<E>, RandomAccess, Cloneable, Serializable
Constructors of Java ArrayList
Methods of Java ArrayList
|
The choice between using an array or an ArrayList
(or similar dynamic array-like data structure) depends on the specific requirements and constraints of your programming task. Both arrays and ArrayLists
have their advantages and trade-offs, and the decision should be based on the context of your application.
Here are some considerations for when to use each:
Use Arrays When:
Fixed Size: Arrays have a fixed size once they are created. If you know the size of the data you need to store and it won't change, arrays can be more memory-efficient and slightly faster than dynamic data structures like
ArrayList
. This is because arrays don't need to dynamically allocate memory.Performance-Critical Applications: In performance-critical applications, where every CPU cycle and memory allocation matters, arrays can offer better performance because they have less overhead compared to dynamic data structures like
ArrayList
.Primitive Types: Arrays are ideal for storing primitive data types (e.g.,
int
,double
,char
) because they don't require boxing and unboxing, which can occur when storing these types in anArrayList
.
Use ArrayList When:
Dynamic Sizing:
ArrayLists
dynamically resize themselves as needed. If you don't know the size of your data in advance or if it may change over time,ArrayLists
are more flexible and convenient.Simpler APIs:
ArrayLists
provide a more user-friendly and feature-rich API compared to arrays. They have built-in methods for adding, removing, and manipulating elements, making your code more readable and maintainable.Generics:
ArrayLists
can store generic types, which provides type safety and avoids the need for explicit casting when retrieving elements.Collections Framework Integration: If you're working with Java's Collections Framework, which includes classes like
List
,Set
, andMap
, usingArrayList
is often more seamless because it implements theList
interface.Interoperability:
ArrayLists
can be easily converted to arrays using thetoArray
method, providing compatibility when working with APIs that require arrays.
In summary, the choice between using an array or an ArrayList
depends on factors such as your application's requirements, performance considerations, the flexibility needed for dynamic data, and the convenience of the provided APIs. Each has its own strengths, and it's important to choose the data structure that best fits your specific use case
- Java LinkedList class can contain duplicate elements.
- Java LinkedList class maintains insertion order.
- Java LinkedList class is non synchronized.
- In Java LinkedList class, manipulation is fast because no shifting needs to occur.
- Java LinkedList class can be used as a list, stack or queue
Doubly Linked List:

Hierarchy of LinkedList class:
Constructors of Java LinkedList
Constructor | Description |
---|---|
LinkedList() | It is used to construct an empty list. |
LinkedList(Collection<? extends E> c) | It is used to construct a list containing the elements of the specified collection, in the order, they are returned by the collection's iterator. |
Methods of Java LinkedList
Method | Description |
---|---|
boolean add(E e) | It is used to append the specified element to the end of a list. |
void add(int index, E element) | It is used to insert the specified element at the specified position index in a list. |
boolean addAll(Collection<? extends E> c) | It is used to append all of the elements in the specified collection to the end of this list, in the order that they are returned by the specified collection's iterator. |
boolean addAll(Collection<? extends E> c) | It is used to append all of the elements in the specified collection to the end of this list, in the order that they are returned by the specified collection's iterator. |
boolean addAll(int index, Collection<? extends E> c) | It is used to append all the elements in the specified collection, starting at the specified position of the list. |
void addFirst(E e) | It is used to insert the given element at the beginning of a list. |
void addLast(E e) | It is used to append the given element to the end of a list. |
void clear() | It is used to remove all the elements from a list. |
Object clone() | It is used to return a shallow copy of an ArrayList. |
boolean contains(Object o) | It is used to return true if a list contains a specified element. |
Iterator<E> descendingIterator() | It is used to return an iterator over the elements in a deque in reverse sequential order. |
E element() | It is used to retrieve the first element of a list. |
E get(int index) | It is used to return the element at the specified position in a list. |
E getFirst() | It is used to return the first element in a list. |
E getLast() | It is used to return the last element in a list. |
int indexOf(Object o) | It is used to return the index in a list of the first occurrence of the specified element, or -1 if the list does not contain any element. |
int lastIndexOf(Object o) | It is used to return the index in a list of the last occurrence of the specified element, or -1 if the list does not contain any element. |
ListIterator<E> listIterator(int index) | It is used to return a list-iterator of the elements in proper sequence, starting at the specified position in the list. |
boolean offer(E e) | It adds the specified element as the last element of a list. |
boolean offerFirst(E e) | It inserts the specified element at the front of a list. |
boolean offerLast(E e) | It inserts the specified element at the end of a list. |
E peek() | It retrieves the first element of a list |
E peekFirst() | It retrieves the first element of a list or returns null if a list is empty. |
E peekLast() | It retrieves the last element of a list or returns null if a list is empty. |
E poll() | It retrieves and removes the first element of a list. |
E pollFirst() | It retrieves and removes the first element of a list, or returns null if a list is empty. |
E pollLast() | It retrieves and removes the last element of a list, or returns null if a list is empty. |
E pop() | It pops an element from the stack represented by a list. |
void push(E e) | It pushes an element onto the stack represented by a list. |
E remove() | It is used to retrieve and removes the first element of a list. |
E remove(int index) | It is used to remove the element at the specified position in a list. |
boolean remove(Object o) | It is used to remove the first occurrence of the specified element in a list. |
E removeFirst() | It removes and returns the first element from a list. |
boolean removeFirstOccurrence(Object o) | It is used to remove the first occurrence of the specified element in a list (when traversing the list from head to tail). |
E removeLast() | It removes and returns the last element from a list. |
boolean removeLastOccurrence(Object o) | It removes the last occurrence of the specified element in a list (when traversing the list from head to tail). |
E set(int index, E element) | It replaces the element at the specified position in a list with the specified element. |
Object[] toArray() | It is used to return an array containing all the elements in a list in proper sequence (from first to the last element). |
<T> T[] toArray(T[] a) | It returns an array containing all the elements in the proper sequence (from first to the last element); the runtime type of the returned array is that of the specified array. |
int size() | It is used to return the number of elements in a list. |
Vector:
Stack:
The stack is the subclass of Vector. It implements the last-in-first-out data structure, i.e., Stack. The stack contains all of the methods of Vector class and also provides its methods like boolean push(), boolean peek(), boolean push(object o), which defines its properties.Stack Methods
push(element):
- Purpose: Adds an element to the top of the stack.
pop():
- Purpose: Removes and returns the top element of the stack. If the stack is empty, this method usually throws an exception (like
EmptyStackException
in Java).
Queue Interface:
Queue interface maintains the first-in-first-out order. It can be defined as an ordered list that is used to hold the elements which are about to be processed. There are various classes like PriorityQueue, Deque, and ArrayDeque which implements the Queue interface.ArrayList | LinkedList |
---|---|
1) ArrayList internally uses a dynamic array to store the elements. | LinkedList internally uses a doubly linked list to store the elements. |
2) Manipulation with ArrayList is slow because it internally uses an array. If any element is removed from the array, all the bits are shifted in memory. | Manipulation with LinkedList is faster than ArrayList because it uses a doubly linked list, so no bit shifting is required in memory. |
3) An ArrayList class can act as a list only because it implements List only. | LinkedList class can act as a list and queue both because it implements List and Deque interfaces. |
4) ArrayList is better for storing and accessing data. | LinkedList is better for manipulating data. |
Java Non-generic Vs. Generic Collection
Java collection framework was non-generic before JDK 1.5. Since 1.5, it is generic.
Java new generic collection allows you to have only one type of object in a collection. Now it is type safe so typecasting is not required at runtime.- Stronger type checks at compile time.
A Java compiler applies strong type checking to generic code and issues errors if the code violates type safety. Fixing compile-time errors is easier than fixing runtime errors, which can be difficult to find.
- Elimination of casts.
The following code snippet without generics requires casting:List list = new ArrayList();
list.add("hello");
String s = (String) list.get(0);
When re-written to use generics, the code does not require casting:List<String> list = new ArrayList<String>();
list.add("hello");
String s = list.get(0); // no cast
- Enabling programmers to implement generic algorithms
Let's see the old non-generic example of creating java collection.
Let's see the new generic example of creating java collection.
In a generic collection, we specify the type in angular braces. Now ArrayList is forced to have the only specified type of objects in it. If you try to add another type of object, it gives compile time error.
List<datatype>collectionname=webdriverpluralmethods();
List collectionName=new ArrayList()Ã non generic format
List<datatype>collectionname=new ArrayList<datatype>(); //generic format
Set<datatype>collectionname=webdriverpluralmethods();
//Set<datatype>collectionname=new implentingclass<datatype>();
Set<datatype>collectionname=new HashSet/LinkedHashSet/TreeSet<datatype>(); ///Generic Format
- Stronger type checks at compile time.
A Java compiler applies strong type checking to generic code and issues errors if the code violates type safety. Fixing compile-time errors is easier than fixing runtime errors, which can be difficult to find. - Elimination of casts.
The following code snippet without generics requires casting:When re-written to use generics, the code does not require casting:List list = new ArrayList(); list.add("hello"); String s = (String) list.get(0);
List<String> list = new ArrayList<String>(); list.add("hello"); String s = list.get(0); // no cast
- Enabling programmers to implement generic algorithms
Set Interface:
Java HashSet

- HashSet stores the elements by using a mechanism called hashing.
- HashSet contains unique elements only.
- HashSet allows null value.
- HashSet class is non synchronized.
- HashSet doesn't maintain the insertion order. Here, elements are inserted on the basis of their hashcode.
- HashSet is the best approach for search operations.
- The initial default capacity of HashSet is 16, and the load factor is 0.75.
HashSet class declaration
java.util.HashSet class.
Constructors of Java HashSet class
SN | Constructor | Description |
---|---|---|
1) | HashSet() | It is used to construct a default HashSet. |
2) | HashSet(int capacity) | It is used to initialize the capacity of the hash set to the given integer value capacity. The capacity grows automatically as elements are added to the HashSet. |
3) | HashSet(int capacity, float loadFactor) | It is used to initialize the capacity of the hash set to the given integer value capacity and the specified load factor. |
4) | HashSet(Collection<? extends E> c) | It is used to initialize the hash set by using the elements of the collection c. |
Methods of Java HashSet class
Various methods of Java HashSet class are as follows:
SN | Modifier & Type | Method | Description |
---|---|---|---|
1) | boolean | add(E e) | It is used to add the specified element to this set if it is not already present. |
2) | void | clear() | It is used to remove all of the elements from the set. |
3) | object | clone() | It is used to return a shallow copy of this HashSet instance: the elements themselves are not cloned. |
4) | boolean | contains(Object o) | It is used to return true if this set contains the specified element. |
5) | boolean | isEmpty() | It is used to return true if this set contains no elements. |
6) | Iterator<E> | iterator() | It is used to return an iterator over the elements in this set. |
7) | boolean | remove(Object o) | It is used to remove the specified element from this set if it is present. |
8) | int | size() | It is used to return the number of elements in the set. |
9) | Spliterator<E> | spliterator() | It is used to create a late-binding and fail-fast Spliterator over the elements in the set. |
LinkedHashSet:
LinkedHashSet class represents the LinkedList implementation of Set Interface. It extends the HashSet class and implements Set interface. Like HashSet, It also contains unique elements. It maintains the insertion order and permits null elements.
TreeSet:Java TreeSet class implements the Set interface that uses a tree for storage. Like HashSet, TreeSet also contains unique elements. However, the access and retrieval time of TreeSet is quite fast. The elements in TreeSet stored in ascending order.
Convert Set into List:
List<String>list=new ArrayList<String>(set);
Covert List into Set collection: Set<datatype>collectioName=new HashSet<datatype>(listCollectionName);
Difference between List and Set:
- A list can contain duplicate elements whereas Set contains unique elements only.
- List is ordered collection where as Set is UnOrdered Collection
- List Elements cane be accessed uisng Index. Whereas Set collection elements cannot be accessed using index
- List is implemented by ArrayList,LinkedinList & Vector where as Set is implemented by HashSet,LinkedinHashSet & treeSet classes
- A list can contain duplicate elements whereas Set contains unique elements only.
- List is ordered collection where as Set is UnOrdered Collection
- List Elements cane be accessed uisng Index. Whereas Set collection elements cannot be accessed using index
- List is implemented by ArrayList,LinkedinList & Vector where as Set is implemented by HashSet,LinkedinHashSet & treeSet classes
Difference between Iterator and ListIterator?
- Iterator can traverse in forward direction only whereas ListIterator can be used to traverse in both directions.
- ListIterator inherits from Iterator interface and comes with extra functions like adding an
- element,replacing an element,getting index position for previous and next elements
- We can use Iterator to traverse Set and List collections whereas ListIterator can be used with Lists only
- Iterator can traverse in forward direction only whereas ListIterator can be used to traverse in both directions.
- ListIterator inherits from Iterator interface and comes with extra functions like adding an
- element,replacing an element,getting index position for previous and next elements
- We can use Iterator to traverse Set and List collections whereas ListIterator can be used with Lists only
How to Iterate Collection:
1)By Iterator interface. Iterator it=collectionname.iterator(); While(it.hasNext()){ System.out.println(it.next()); }
2)By for-each loop. //for(datatype varaiblename:collectionname){statements+logic}
3)By normal for loop for(initialization;condition;increment/decrement){statements+logic}
EXERCISES:1. Write a Java program to create a new array list, add some colors (string) and print out the collection.2)Write a Java program to iterate through all elements in a array list.3) Write a Java program to insert an element into the array list at the first position4)Write a Java program to retrieve an element (at a specified index) from a given array list5)Write a Java program to update specific arraylist element by given element.6)Write a Java program to remove the third element from a array list.7)Write a Java program to search an element in a array list8)Write a Java program to sort a given array list.9)Write a Java program to copy one array list into another10)Write a Java program to empty an array list11)Write a Java program to test an array list is empty or not-----------------------------------------------------------------------------------------------Interview Program 1)Write a program to arrange even numbers at the beginning and then odd numbers of the * given array int[] arr={1,2,5,4,7,8,11,20};Hint:Use ArrayList methods add() and addAll()-----------------------------------------------------------------------------------------------Interview Program 2)Write a java program to print union of two array elements and common elements from two given arraysint[] arr1= {80,10,15,2,35,60}; int[] arr2= {35,80,60,20,75};Hint: Create HashSet2)iterate first array and add all the elements to set collection3)iterate second array and add all the elements to set collection4)Then print the set collection for union elements
PartII) Common elements from both the Arrays1)create a hashset2)iterate first array and add all the elements to set collection3)iterate the second array and check array element is present in set collection then print that array element----------------------------------------------------------------------INTRVIEW EXERCISE3)Write a program to find duplicate elements in given arrayint[] arr= {2,1,3,5,3,2};Hint) 1)Create Set Collection2)Iterate the array and check HashSet contains array element using contains() if true print the arrayelement--------------------------------------------------------------import java.util.HashSet;import java.util.Set;
public class Solution { public int[][] setZeroes(int[][] A) { Set<Integer> rows = new HashSet<>(); Set<Integer> cols = new HashSet<>();
// Find rows and columns with zeros for (int i = 0; i < A.length; i++) { for (int j = 0; j < A[0].length; j++) { if (A[i][j] == 0) { rows.add(i); cols.add(j); } } }
// Set zeros for rows for (int i : rows) { for (int j = 0; j < A[0].length; j++) { A[i][j] = 0; } }
// Set zeros for columns for (int j : cols) { for (int i = 0; i < A.length; i++) { A[i][j] = 0; } }
return A; }
public static void main(String[] args) { Solution solution = new Solution(); int[][] A1 = { {1, 0, 1}, {1, 1, 1}, {1, 1, 1} }; int[][] A2 = { {1, 0, 1}, {1, 1, 1}, {1, 0, 1} };
int[][] result1 = solution.setZeroes(A1); int[][] result2 = solution.setZeroes(A2);
System.out.println("Output 1:"); for (int[] row : result1) { for (int cell : row) { System.out.print(cell + " "); } System.out.println(); }
System.out.println("Output 2:"); for (int[] row : result2) { for (int cell : row) { System.out.print(cell + " "); } System.out.println(); } }}=======================================================
---------------------
Java Map Interface:A map contains values on the basis of key, i.e. key and value pair. Each key and value pair is known as an entry. A Map contains unique keys.A Map is useful if you have to search, update or delete elements on the basis of a key.
Java Map Hierarchy
There are two interfaces for implementing Map in java: Map and SortedMap, and three classes: HashMap, LinkedHashMap, and TreeMap. The hierarchy of Java Map is given below:

A Map doesn't allow duplicate keys, but you can have duplicate values. HashMap and LinkedHashMap allow null keys and values, but TreeMap doesn't allow any null key or value.A Map can't be traversed, so you need to convert it into Set using keySet() or entrySet() method.Class Description HashMap HashMap is the implementation of Map, but it doesn't maintain any order. LinkedHashMap LinkedHashMap is the implementation of Map. It inherits HashMap class. It maintains insertion order. TreeMap TreeMap is the implementation of Map and SortedMap. It maintains ascending order.

Class | Description |
---|---|
HashMap | HashMap is the implementation of Map, but it doesn't maintain any order. |
LinkedHashMap | LinkedHashMap is the implementation of Map. It inherits HashMap class. It maintains insertion order. |
TreeMap | TreeMap is the implementation of Map and SortedMap. It maintains ascending order. |
Useful methods of Map interface
Method Description V put(Object key, Object value) It is used to insert an entry in the map. void putAll(Map map) It is used to insert the specified map in the map. V putIfAbsent(K key, V value) It inserts the specified value with the specified key in the map only if it is not already specified. V remove(Object key) It is used to delete an entry for the specified key. boolean remove(Object key, Object value) It removes the specified values with the associated specified keys from the map. Set keySet() It returns the Set view containing all the keys. Set<Map.Entry<K,V>> entrySet() It returns the Set view containing all the keys and values. void clear() It is used to reset the map. V compute(K key, BiFunction<? super K,? super V,? extends V> remappingFunction) It is used to compute a mapping for the specified key and its current mapped value (or null if there is no current mapping). V computeIfAbsent(K key, Function<? super K,? extends V> mappingFunction) It is used to compute its value using the given mapping function, if the specified key is not already associated with a value (or is mapped to null), and enters it into this map unless null. V computeIfPresent(K key, BiFunction<? super K,? super V,? extends V> remappingFunction) It is used to compute a new mapping given the key and its current mapped value if the value for the specified key is present and non-null. boolean containsValue(Object value) This method returns true if some value equal to the value exists within the map, else return false. boolean containsKey(Object key) This method returns true if some key equal to the key exists within the map, else return false. boolean equals(Object o) It is used to compare the specified Object with the Map. void forEach(BiConsumer<? super K,? super V> action) It performs the given action for each entry in the map until all entries have been processed or the action throws an exception. V get(Object key) This method returns the object that contains the value associated with the key. V getOrDefault(Object key, V defaultValue) It returns the value to which the specified key is mapped, or defaultValue if the map contains no mapping for the key. int hashCode() It returns the hash code value for the Map boolean isEmpty() This method returns true if the map is empty; returns false if it contains at least one key. V merge(K key, V value, BiFunction<? super V,? super V,? extends V> remappingFunction) If the specified key is not already associated with a value or is associated with null, associates it with the given non-null value. V replace(K key, V value) It replaces the specified value for a specified key. boolean replace(K key, V oldValue, V newValue) It replaces the old value with the new value for a specified key. void replaceAll(BiFunction<? super K,? super V,? extends V> function) It replaces each entry's value with the result of invoking the given function on that entry until all entries have been processed or the function throws an exception. Collection values() It returns a collection view of the values contained in the map. int size() This method returns the number of entries in the map.
Method | Description |
---|---|
V put(Object key, Object value) | It is used to insert an entry in the map. |
void putAll(Map map) | It is used to insert the specified map in the map. |
V putIfAbsent(K key, V value) | It inserts the specified value with the specified key in the map only if it is not already specified. |
V remove(Object key) | It is used to delete an entry for the specified key. |
boolean remove(Object key, Object value) | It removes the specified values with the associated specified keys from the map. |
Set keySet() | It returns the Set view containing all the keys. |
Set<Map.Entry<K,V>> entrySet() | It returns the Set view containing all the keys and values. |
void clear() | It is used to reset the map. |
V compute(K key, BiFunction<? super K,? super V,? extends V> remappingFunction) | It is used to compute a mapping for the specified key and its current mapped value (or null if there is no current mapping). |
V computeIfAbsent(K key, Function<? super K,? extends V> mappingFunction) | It is used to compute its value using the given mapping function, if the specified key is not already associated with a value (or is mapped to null), and enters it into this map unless null. |
V computeIfPresent(K key, BiFunction<? super K,? super V,? extends V> remappingFunction) | It is used to compute a new mapping given the key and its current mapped value if the value for the specified key is present and non-null. |
boolean containsValue(Object value) | This method returns true if some value equal to the value exists within the map, else return false. |
boolean containsKey(Object key) | This method returns true if some key equal to the key exists within the map, else return false. |
boolean equals(Object o) | It is used to compare the specified Object with the Map. |
void forEach(BiConsumer<? super K,? super V> action) | It performs the given action for each entry in the map until all entries have been processed or the action throws an exception. |
V get(Object key) | This method returns the object that contains the value associated with the key. |
V getOrDefault(Object key, V defaultValue) | It returns the value to which the specified key is mapped, or defaultValue if the map contains no mapping for the key. |
int hashCode() | It returns the hash code value for the Map |
boolean isEmpty() | This method returns true if the map is empty; returns false if it contains at least one key. |
V merge(K key, V value, BiFunction<? super V,? super V,? extends V> remappingFunction) | If the specified key is not already associated with a value or is associated with null, associates it with the given non-null value. |
V replace(K key, V value) | It replaces the specified value for a specified key. |
boolean replace(K key, V oldValue, V newValue) | It replaces the old value with the new value for a specified key. |
void replaceAll(BiFunction<? super K,? super V,? extends V> function) | It replaces each entry's value with the result of invoking the given function on that entry until all entries have been processed or the function throws an exception. |
Collection | It returns a collection view of the values contained in the map. |
int size() | This method returns the number of entries in the map. |
Map.Entry Interface:Entry is the subinterface of Map. So we will be accessed it by Map.Entry name. It returns a collection-view of the map, whose elements are of this class. It provides methods to get key and value.
Methods of Map.Entry interface
Method Description K getKey() It is used to obtain a key. V getValue() It is used to obtain value. int hashCode() It is used to obtain hashCode.
Method | Description |
---|---|
K getKey() | It is used to obtain a key. |
V getValue() | It is used to obtain value. |
int hashCode() | It is used to obtain hashCode. |
Java HashMap class
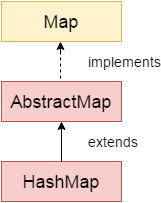
Java HashMap class implements the map interface by using a hash table. It inherits AbstractMap class and implements Map interface.
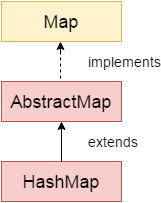
Points to remember
- Java HashMap class contains values based on the key.
- Java HashMap class contains only unique keys.
- Java HashMap class may have one null key and multiple null values.
- Java HashMap class is non synchronized.
- Java HashMap class maintains no order.
- The initial default capacity of Java HashMap class is 16 with a load factor of 0.75.
- Java HashMap class contains values based on the key.
- Java HashMap class contains only unique keys.
- Java HashMap class may have one null key and multiple null values.
- Java HashMap class is non synchronized.
- Java HashMap class maintains no order.
- The initial default capacity of Java HashMap class is 16 with a load factor of 0.75.
Hierarchy of HashMap class
As shown in the above figure, HashMap class extends AbstractMap class and implements Map interface.
HashMap class declaration
Let's see the declaration for java.util.HashMap class.
HashMap class Parameters
Let's see the Parameters for java.util.HashMap class.- K: It is the type of keys maintained by this map.
- V: It is the type of mapped values.
- K: It is the type of keys maintained by this map.
- V: It is the type of mapped values.
Constructors of Java HashMap class
Constructor Description HashMap() It is used to construct a default HashMap. HashMap(Map<? extends K,? extends V> m) It is used to initialize the hash map by using the elements of the given Map object m. HashMap(int capacity) It is used to initializes the capacity of the hash map to the given integer value, capacity. HashMap(int capacity, float loadFactor) It is used to initialize both the capacity and load factor of the hash map by using its arguments.
Constructor | Description |
---|---|
HashMap() | It is used to construct a default HashMap. |
HashMap(Map<? extends K,? extends V> m) | It is used to initialize the hash map by using the elements of the given Map object m. |
HashMap(int capacity) | It is used to initializes the capacity of the hash map to the given integer value, capacity. |
HashMap(int capacity, float loadFactor) | It is used to initialize both the capacity and load factor of the hash map by using its arguments. |
Methods of Java HashMap class
Method Description void clear() It is used to remove all of the mappings from this map. boolean isEmpty() It is used to return true if this map contains no key-value mappings. Object clone() It is used to return a shallow copy of this HashMap instance: the keys and values themselves are not cloned. Set entrySet() It is used to return a collection view of the mappings contained in this map. Set keySet() It is used to return a set view of the keys contained in this map. V put(Object key, Object value) It is used to insert an entry in the map. void putAll(Map map) It is used to insert the specified map in the map. V putIfAbsent(K key, V value) It inserts the specified value with the specified key in the map only if it is not already specified. V remove(Object key) It is used to delete an entry for the specified key. boolean remove(Object key, Object value) It removes the specified values with the associated specified keys from the map. V compute(K key, BiFunction<? super K,? super V,? extends V> remappingFunction) It is used to compute a mapping for the specified key and its current mapped value (or null if there is no current mapping). V computeIfAbsent(K key, Function<? super K,? extends V> mappingFunction) It is used to compute its value using the given mapping function, if the specified key is not already associated with a value (or is mapped to null), and enters it into this map unless null. V computeIfPresent(K key, BiFunction<? super K,? super V,? extends V> remappingFunction) It is used to compute a new mapping given the key and its current mapped value if the value for the specified key is present and non-null. boolean containsValue(Object value) This method returns true if some value equal to the value exists within the map, else return false. boolean containsKey(Object key) This method returns true if some key equal to the key exists within the map, else return false. boolean equals(Object o) It is used to compare the specified Object with the Map. void forEach(BiConsumer<? super K,? super V> action) It performs the given action for each entry in the map until all entries have been processed or the action throws an exception. V get(Object key) This method returns the object that contains the value associated with the key. V getOrDefault(Object key, V defaultValue) It returns the value to which the specified key is mapped, or defaultValue if the map contains no mapping for the key. boolean isEmpty() This method returns true if the map is empty; returns false if it contains at least one key. V merge(K key, V value, BiFunction<? super V,? super V,? extends V> remappingFunction) If the specified key is not already associated with a value or is associated with null, associates it with the given non-null value. V replace(K key, V value) It replaces the specified value for a specified key. boolean replace(K key, V oldValue, V newValue) It replaces the old value with the new value for a specified key. void replaceAll(BiFunction<? super K,? super V,? extends V> function) It replaces each entry's value with the result of invoking the given function on that entry until all entries have been processed or the function throws an exception. Collection<V> values() It returns a collection view of the values contained in the map. int size() This method returns the number of entries in the map.
Method | Description |
---|---|
void clear() | It is used to remove all of the mappings from this map. |
boolean isEmpty() | It is used to return true if this map contains no key-value mappings. |
Object clone() | It is used to return a shallow copy of this HashMap instance: the keys and values themselves are not cloned. |
Set entrySet() | It is used to return a collection view of the mappings contained in this map. |
Set keySet() | It is used to return a set view of the keys contained in this map. |
V put(Object key, Object value) | It is used to insert an entry in the map. |
void putAll(Map map) | It is used to insert the specified map in the map. |
V putIfAbsent(K key, V value) | It inserts the specified value with the specified key in the map only if it is not already specified. |
V remove(Object key) | It is used to delete an entry for the specified key. |
boolean remove(Object key, Object value) | It removes the specified values with the associated specified keys from the map. |
V compute(K key, BiFunction<? super K,? super V,? extends V> remappingFunction) | It is used to compute a mapping for the specified key and its current mapped value (or null if there is no current mapping). |
V computeIfAbsent(K key, Function<? super K,? extends V> mappingFunction) | It is used to compute its value using the given mapping function, if the specified key is not already associated with a value (or is mapped to null), and enters it into this map unless null. |
V computeIfPresent(K key, BiFunction<? super K,? super V,? extends V> remappingFunction) | It is used to compute a new mapping given the key and its current mapped value if the value for the specified key is present and non-null. |
boolean containsValue(Object value) | This method returns true if some value equal to the value exists within the map, else return false. |
boolean containsKey(Object key) | This method returns true if some key equal to the key exists within the map, else return false. |
boolean equals(Object o) | It is used to compare the specified Object with the Map. |
void forEach(BiConsumer<? super K,? super V> action) | It performs the given action for each entry in the map until all entries have been processed or the action throws an exception. |
V get(Object key) | This method returns the object that contains the value associated with the key. |
V getOrDefault(Object key, V defaultValue) | It returns the value to which the specified key is mapped, or defaultValue if the map contains no mapping for the key. |
boolean isEmpty() | This method returns true if the map is empty; returns false if it contains at least one key. |
V merge(K key, V value, BiFunction<? super V,? super V,? extends V> remappingFunction) | If the specified key is not already associated with a value or is associated with null, associates it with the given non-null value. |
V replace(K key, V value) | It replaces the specified value for a specified key. |
boolean replace(K key, V oldValue, V newValue) | It replaces the old value with the new value for a specified key. |
void replaceAll(BiFunction<? super K,? super V,? extends V> function) | It replaces each entry's value with the result of invoking the given function on that entry until all entries have been processed or the function throws an exception. |
Collection<V> values() | It returns a collection view of the values contained in the map. |
int size() | This method returns the number of entries in the map. |
If hashmap is available then why do you need HashSet?
HashMap
and HashSet
serve different purposes and have distinct use cases, even though they both use hash-based data structures.
Here's a brief explanation of each and when you might choose one over the other:
HashMap:
- A
HashMap
is a key-value data structure that allows you to store and retrieve values associated with unique keys. - It associates each key with a specific value, making it suitable for mapping relationships between keys and values.
- Common use cases for
HashMap
include storing configuration settings, caching, and representing relationships in data structures like graphs (using adjacency lists). - When you need to associate values with specific identifiers or keys,
HashMap
is a good choice.
Example in Java:
javaMap<String, Integer> ageMap = new HashMap<>(); ageMap.put("Alice", 25); ageMap.put("Bob", 30); int aliceAge = ageMap.get("Alice"); // Retrieves the age of A
- A
HashSet:
- A
HashSet
is an unordered collection of unique elements, typically used to ensure that a collection contains no duplicate values. - It is often used when you want to check for the presence of an element in a collection without regard to its position.
- Common use cases for
HashSet
include implementing sets and ensuring uniqueness in a collection of items. - When you need to maintain a collection of unique elements or efficiently check for the presence of an element,
HashSet
is a suitable choice.
Example in Java:
Set<String> uniqueNames = new HashSet<>(); uniqueNames.add("Alice"); uniqueNames.add("Bob"); boolean containsAlice = uniqueNames.contains("Alice"); // Checks if "Alice" is in the set
HashMap
and HashSet
use hash-based data structures, they have different purposes. HashMap
is used for mapping keys to values, while HashSet
is used for maintaining collections of unique elements. The choice between them depends on the specific requirements of your data and the operations you need to perform.HOW HASHMAP WORKS INTERNALLY?
JVM will take the put method key value and calculate the hashcode of key and then in step2 find the index of that hashcode
JVM checks if same key is present in the bucket or not using equals() if same key is present then jvm will update the existing key with new value.
How Searching will happen in linkedlist is one by one node will search that is the drawback of linkedlist. It reduces the performance
How we will get key value in HashMap describes below picture
int b = a ;
System.out.println(a); -> o/p - 20
System.out.println(b); -> o/p - 20
a=30;
System.out.println(a); -> o/p - 30
System.out.println(b); -> o/p - 20
But when we do same operation in HashMaps
Map<String, Integer> map1 = new HashMap<>();
map1.put(“value”, 25);
Map<String, Integer> map2 = new HashMap<>();
map1=map2;
System.out.println(map1); -> o/p - value,25
System.out.println(map2); -> o/p - 20 - value,25
Now if we update this to -
map1.put(“value”, 42)
System.out.println(map1); -> o/p - value,42
System.out.println(map2); -> o/p - 20 - value,42
Why does this happen??
HashMap
. Let's break down the behavior step by step- Initially, you create two
HashMap
instances,map1
andmap2
. - You put a key-value pair ("value", 25) into
map1
. - Then, you make
map1
reference the same object asmap2
. This means bothmap1
andmap2
now refer to the sameHashMap
object, effectively discarding the originalmap1
. - When you print
map1
andmap2
, they both refer to the sameHashMap
object, so you see the same content ("value", 25).
Now, let's look at the update:
- You update the
map1
by putting a new key-value pair ("value", 42). Sincemap1
andmap2
both reference the sameHashMap
object, the change is reflected in both references. - When you print
map1
andmap2
again, they both reference the same updatedHashMap
object, so you see the updated content ("value", 42).
This behavior is because map1
and map2
are references to the same HashMap
object in memory. Changing the content through one reference affects the object, and any other references to that object will see the changes.
In contrast, in your initial example with integers (int
), you are dealing with primitive data types. When you assign b = a
, you are making a copy of the value of a
. Changing a
afterward does not affect b
because b
holds a separate copy of the value. This is different from reference types like HashMap
, where the references point to the same object in memory.
=====================================
Difference between HashMap and Hashtable
HashMap | Hashtable |
---|---|
1) HashMap is non synchronized. It is not-thread safe and can't be shared between many threads without proper synchronization code. | Hashtable is synchronized. It is thread-safe and can be shared with many threads. |
2) HashMap allows one null key and multiple null values. | Hashtable doesn't allow any null key or value. |
3) HashMap is a new class introduced in JDK 1.2. | Hashtable is a legacy class. |
4) HashMap is fast. | Hashtable is slow. |
5) We can make the HashMap as synchronized by calling this code Map m = Collections.synchronizedMap(hashMap); | Hashtable is internally synchronized and can't be unsynchronized. |
6) HashMap is traversed by Iterator. | Hashtable is traversed by Enumerator and Iterator. |
7) Iterator in HashMap is fail-fast. | Enumerator in Hashtable is not fail-fast. |
8) HashMap inherits AbstractMap class. | Hashtable inherits Dictionary class. |
Sorting in Collection
We can sort the elements of:
- String objects
- Wrapper class objects
- User-defined class objects
Collections class provides static methods for sorting the elements of a collection. If collection elements are of a Set type, we can use TreeSet. However, we cannot sort the elements of List. Collections class provides methods for sorting the elements of List type elements. |
Method of Collections class for sorting List elements
public void sort(List list): is used to sort the elements of List. List elements must be of the Comparable type.
Note: String class and Wrapper classes implement the Comparable interface. So if you store the objects of string or wrapper classes, it will be Comparable
Java Comparable interface
Java Comparable interface is used to order the objects of the user-defined class. This interface is found in java.lang package and contains only one method named compareTo(Object). It provides a single sorting sequence only, i.e., you can sort the elements on the basis of single data member only. For example, it may be rollno, name, age or anything else.
compareTo(Object obj) method
public int compareTo(Object obj): It is used to compare the current object with the specified object. It returns
- positive integer, if the current object is greater than the specified object.
- negative integer, if the current object is less than the specified object.
- zero, if the current object is equal to the specified object.
Java Comparator interface
Java Comparator interface is used to order the objects of a user-defined class.
This interface is found in java.util package and contains 2 methods compare(Object obj1,Object obj2) and equals(Object element).
It provides multiple sorting sequences, i.e., you can sort the elements on the basis of any data member, for example, rollno, name, age or anything else.
Methods of Java Comparator Interface
Method | Description |
---|---|
public int compare(Object obj1, Object obj2) | It compares the first object with the second object. |
public boolean equals(Object obj) | It is used to compare the current object with the specified object. |
public boolean equals(Object obj) | It is used to compare the current object with the specified object. |
Collections class
Collections class provides static methods for sorting the elements of a collection. If collection elements are of Set or Map, we can use TreeSet or TreeMap. However, we cannot sort the elements of List. Collections class provides methods for sorting the elements of List type elements also.
Method of Collections class for sorting List elements
public void sort(List list, Comparator c): is used to sort the elements of List by the given Comparator
Difference between Comparable and Comparator
Comparable | Comparator |
---|---|
1) Comparable provides a single sorting sequence. In other words, we can sort the collection on the basis of a single element such as id, name, and price. | The Comparator provides multiple sorting sequences. In other words, we can sort the collection on the basis of multiple elements such as id, name, and price etc. |
2) Comparable affects the original class, i.e., the actual class is modified. | Comparator doesn't affect the original class, i.e., the actual class is not modified. |
3) Comparable provides compareTo() method to sort elements. | Comparator provides compare() method to sort elements. |
4) Comparable is present in java.lang package. | A Comparator is present in the java.util package. |
5) We can sort the list elements of Comparable type by Collections.sort(List) method. | We can sort the list elements of Comparator type by Collections.sort(List, Comparator) method. |
Difference between ArrayList and Vector
ArrayList | Vector |
---|---|
1) ArrayList is not synchronized. | Vector is synchronized. |
2) ArrayList increments 50% of current array size if the number of elements exceeds from its capacity. | Vector increments 100% means doubles the array size if the total number of elements exceeds than its capacity. |
3) ArrayList is not a legacy class. It is introduced in JDK 1.2. | Vector is a legacy class. |
4) ArrayList is fast because it is non-synchronized. | Vector is slow because it is synchronized, i.e., in a multithreading environment, it holds the other threads in runnable or non-runnable state until current thread releases the lock of the object. |
5) ArrayList uses the Iterator interface to traverse the elements. | A Vector can use the Iterator interface or Enumeration interface to traverse the elements. |
================================================
Differences between Collection and Collections
Arrays vs Collection
====================================
Properties class in Java
An Advantage of the properties file
Constructors of Properties class
Method | Description |
---|---|
Properties() | It creates an empty property list with no default values. |
Properties(Properties defaults) | It creates an empty property list with the specified defaults. |
Methods of Properties class
Method | Description |
---|---|
public void load(Reader r) | It loads data from the Reader object. |
public void load(InputStream is) | It loads data from the InputStream object |
public void loadFromXML(InputStream in) | It is used to load all of the properties represented by the XML document on the specified input stream into this properties table. |
public String getProperty(String key) | It returns value based on the key. |
public String getProperty(String key, String defaultValue) | It searches for the property with the specified key. |
public void setProperty(String key, String value) | It calls the put method of Hashtable. |
public void list(PrintStream out) | It is used to print the property list out to the specified output stream. |
public void list(PrintWriter out)) | It is used to print the property list out to the specified output stream. |
public Enumeration<?> propertyNames()) | It returns an enumeration of all the keys from the property list. |
public Set<String> stringPropertyNames() | It returns a set of keys in from property list where the key and its corresponding value are strings. |
public void store(Writer w, String comment) | It writes the properties in the writer object. |
public void store(OutputStream os, String comment) | It writes the properties in the OutputStream object. |
public void storeToXML(OutputStream os, String comment) | It writes the properties in the writer object for generating XML document. |
public void storeToXML(Writer w, String comment, String encoding) | It writes the properties in the writer object for generating XML document with the specified encoding. |
Difference Between Collections Vs Streams In Java :
Collections | Streams |
Collections are mainly used to store and group the data. | Streams are mainly used to perform operations on data. |
You can add or remove elements from collections. | You can’t add or remove elements from streams. |
Collections have to be iterated externally. | Streams are internally iterated. |
Collections can be traversed multiple times. | Streams are traversable only once. |
Collections are eagerly constructed. | Streams are lazily constructed. |
Ex : List, Set, Map… | Ex : filtering, mapping, matching… |
Collections Programs:
Sets Union Intersection Difference and Complement
Intersection: Set of members that belong to the first set "and" the second set.
Difference: Set of members that belong to the first set "and not" the second set.
Complement: Set of members that belong to the second (universal) set "and not" the first set.
Given the following sets:
A={2,4,6,8,10}
B={1,2,3,4,5}
Union: A∪B = {1,2,3,4,5,6,8,10}
Intersection: A∩B = {2,4}
Difference: A-B = {6,8,10}
Complement: AB = {1,3,5}
No comments:
Post a Comment
Note: Only a member of this blog may post a comment.