The Exception Handling in Java is one of the powerful mechanism to handle the runtime errors so that normal flow of the application can be maintained.
Java finally block is always executed whether exception is handled or not.
Java finally block follows try or catch block.
We can throw either checked or uncheked exception in java by throw keyword. The throw keyword is mainly used to throw custom exception.
Exception Handling is mainly used to handle the checked exceptions. If there occurs any unchecked exception such as NullPointerException, it is programmers fault that he is not performing check up before the code being used.
What is an Exception?
An exception is an unwanted or unexpected event, which occurs during the execution of a program i.e at run time, that disrupts the normal flow of the program’s instructions.
Error vs Exception
Error: An Error indicates serious problem that a reasonable application should not try to catch.
Exception: Exception indicates conditions that a reasonable application might try to catch.
Exception: Exception indicates conditions that a reasonable application might try to catch.
When an exception occurs program execution gets terminated. In such cases we get a system generated error message. The good thing about exceptions is that they can be handled in Java. By handling the exceptions we can provide a meaningful message to the user about the issue rather than a system generated message, which may not be understandable to a user.
Why an exception occurs?
There can be several reasons that can cause a program to throw exception. For example: Opening a non-existing file in your program, Network connection problem, bad input data provided by user etc.
Hierarchy of Java Exception classes
The java.lang.Throwable class is the root class of Java Exception hierarchy which is inherited by two subclasses: Exception and Error. A hierarchy of Java Exception classes are given below:
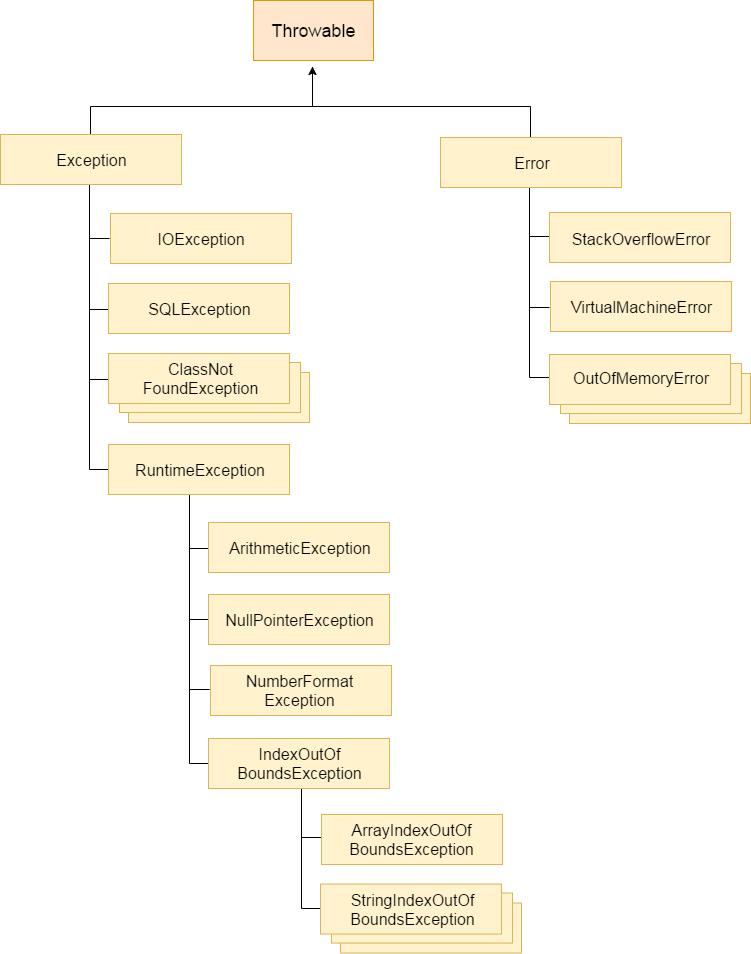
Difference between Checked and Unchecked Exceptions
1) Checked Exception
The classes which directly inherit Throwable class except RuntimeException and Error are known as checked exceptions e.g. IOException, SQLException etc. Checked exceptions are checked at compile-time.
2) Unchecked Exception
The classes which inherit RuntimeException are known as unchecked exceptions e.g. ArithmeticException, NullPointerException, ArrayIndexOutOfBoundsException etc. Unchecked exceptions are not checked at compile-time, but they are checked at runtime
Common Scenarios of Java Exceptions
There are given some scenarios where unchecked exceptions may occur. They are as follows:
1) A scenario where ArithmeticException occurs
If we divide any number by zero, there occurs an ArithmeticException.
2) A scenario where NullPointerException occurs
If we have a null value in any variable, performing any operation on the variable throws a NullPointerException.
3) A scenario where NumberFormatException occurs
The wrong formatting of any value may occur NumberFormatException. Suppose I have a string variable that has characters, converting this variable into digit will occur NumberFormatException.
4) A scenario where ArrayIndexOutOfBoundsException occurs
If you are inserting any value in the wrong index, it would result in ArrayIndexOutOfBoundsException as shown below:
Java try-catch block
Java try block
Java try block is used to enclose the code that might throw an exception. It must be used within the method.
If an exception occurs at the particular statement of try block, the rest of the block code will not execute. So, it is recommended not to keeping the code in try block that will not throw an exception.
Java try block must be followed by either catch or finally block.
Syntax of Java try-catch
Syntax of try catch in java
try { //statements that may cause an exception } catch (exception(type) e(object)) { //error handling code }
Syntax of try-finally block
Java catch block
Java catch block is used to handle the Exception by declaring the type of exception within the parameter. The declared exception must be the parent class exception ( i.e., Exception) or the generated exception type. However, the good approach is to declare the generated type of exception.
The catch block must be used after the try block only. You can use multiple catch block with a single try block.
Java Multi-catch block
A try block can be followed by one or more catch blocks. Each catch block must contain a different exception handler. So, if you have to perform different tasks at the occurrence of different exceptions, use java multi-catch block.Points to remember
- At a time only one exception occurs and at a time only one catch block is executed.
- All catch blocks must be ordered from most specific to most general, i.e. catch for ArithmeticException must come before catch for Exception.
Example 1
Let's see a simple example of java multi-catch block.Java finally block
Java finally block is a block that is used to execute important code such as closing connection, stream etc.Java finally block is always executed whether exception is handled or not.
Java finally block follows try or catch block.
Syntax of Finally block
try { //Statements that may cause an exception } catch { //Handling exception } finally { //Statements to be executed }
A Simple Example of finally block
Here you can see that the exception occurred in try block which has been handled in catch block, after that finally block got executed.
class Example { public static void main(String args[]) { try{ int num=121/0; System.out.println(num); } catch(ArithmeticException e){ System.out.println("Number should not be divided by zero"); } /* Finally block will always execute * even if there is no exception in try block */ finally{ System.out.println("This is finally block"); } System.out.println("Out of try-catch-finally"); } }
Output:
Number should not be divided by zero This is finally block Out of try-catch-finally
Why use java finally
- Finally block in java can be used to put "cleanup" code such as closing a file, closing connection etc
Cases when the finally block doesn’t execute
The circumstances that prevent execution of the code in a finally block are:
– The death of a Thread
– Using of the System. exit() method.
– Due to an exception arising in the finally block.
– The death of a Thread
– Using of the System. exit() method.
– Due to an exception arising in the finally block.
Finally and Close()
close() statement is used to close all the open streams in a program. Its a good practice to use close() inside finally block. Since finally block executes even if exception occurs so you can be sure that all input and output streams are closed properly regardless of whether the exception occurs or not.
Java throw keyword
The Java throw keyword is used to explicitly throw an exception.We can throw either checked or uncheked exception in java by throw keyword. The throw keyword is mainly used to throw custom exception.
Syntax of throw keyword:
throw new exception_class("error message");
For example:
throw new ArithmeticException("dividing a number by 5 is not allowed in this program");
Example of throw keyword
Lets say we have a requirement where we we need to only register the students when their age is less than 12 and weight is less than 40, if any of the condition is not met then the user should get an ArithmeticException with the warning message “Student is not eligible for registration”. We have implemented the logic by placing the code in the method that checks student eligibility if the entered student age and weight doesn’t met the criteria then we throw the exception using throw keyword.
/* In this program we are checking the Student age * if the student age<12 and weight <40 then our program * should return that the student is not eligible for registration. */ public class ThrowExample { static void checkEligibilty(int stuage, int stuweight){ if(stuage<12 && stuweight<40) { throw new ArithmeticException("Student is not eligible for registration"); } else { System.out.println("Student Entry is Valid!!"); } } public static void main(String args[]){ System.out.println("Welcome to the Registration process!!"); checkEligibilty(10, 39); System.out.println("Have a nice day.."); } }
Output:
Welcome to the Registration process!!Exception in thread "main" java.lang.ArithmeticException: Student is not eligible for registration at beginnersbook.com.ThrowExample.checkEligibilty(ThrowExample.java:9) at beginnersbook.com.ThrowExample.main(ThrowExample.java:18)
============================================================
Java throws keyword
The Java throws keyword is used to declare an exception. It gives an information to the programmer that there may occur an exception so it is better for the programmer to provide the exception handling code so that normal flow can be maintained.Exception Handling is mainly used to handle the checked exceptions. If there occurs any unchecked exception such as NullPointerException, it is programmers fault that he is not performing check up before the code being used.
s we know that there are two types of exception checked and unchecked. Checked exception (compile time) force you to handle them, if you don’t handle them then the program will not compile.
On the other hand unchecked exception (Runtime) doesn’t get checked during compilation. Throws keyword is used for handling checked exceptions . By using throws we can declare multiple exceptions in one go.
On the other hand unchecked exception (Runtime) doesn’t get checked during compilation. Throws keyword is used for handling checked exceptions . By using throws we can declare multiple exceptions in one go.
What is the need of having throws keyword when you can handle exception using try-catch?
Well, thats a valid question. We already know we can handle exceptions using try-catch block.
The throws does the same thing that try-catch does but there are some cases where you would prefer throws over try-catch. For example:
Lets say we have a method
The throws does the same thing that try-catch does but there are some cases where you would prefer throws over try-catch. For example:
Lets say we have a method
myMethod()
that has statements that can throw either ArithmeticException or NullPointerException, in this case you can use try-catch as shown below:public void myMethod() { try { // Statements that might throw an exception } catch (ArithmeticException e) { // Exception handling statements } catch (NullPointerException e) { // Exception handling statements } }
But suppose you have several such methods that can cause exceptions, in that case it would be tedious to write these try-catch for each method. The code will become unnecessary long and will be less-readable.
One way to overcome this problem is by using throws like this: declare the exceptions in the method signature using throws and handle the exceptions where you are calling this method by using try-catch.
Another advantage of using this approach is that you will be forced to handle the exception when you call this method, all the exceptions that are declared using throws, must be handled where you are calling this method else you will get compilation error.
Another advantage of using this approach is that you will be forced to handle the exception when you call this method, all the exceptions that are declared using throws, must be handled where you are calling this method else you will get compilation error.
public void myMethod() throws ArithmeticException, NullPointerException { // Statements that might throw an exception } public static void main(String args[]) { try { myMethod(); } catch (ArithmeticException e) { // Exception handling statements } catch (NullPointerException e) { // Exception handling statements } }
Example of throws Keyword
In this example the method myMethod() is throwing two checked exceptions so we have declared these exceptions in the method signature using throws Keyword. If we do not declare these exceptions then the program will throw a compilation error.
import java.io.*; class ThrowExample { void myMethod(int num)throws IOException, ClassNotFoundException{ if(num==1) throw new IOException("IOException Occurred"); else throw new ClassNotFoundException("ClassNotFoundException"); } } public class Example1{ public static void main(String args[]){ try{ ThrowExample obj=new ThrowExample(); obj.myMethod(1); }catch(Exception ex){ System.out.println(ex); } } }
Output:
java.io.IOException: IOException Occurred
===================================================
Difference between throw and throws in Java
There are many differences between throw and throws keywords. A list of differences between throw and throws are given below:No. | throw | throws |
---|---|---|
1) | Java throw keyword is used to explicitly throw an exception. | Java throws keyword is used to declare an exception. |
2) | Checked exception cannot be propagated using throw only. | Checked exception can be propagated with throws. |
3) | Throw is followed by an instance. | Throws is followed by class. |
4) | Throw is used within the method. | Throws is used with the method signature. |
5) | You cannot throw multiple exceptions. | You can declare multiple exceptions e.g. public void method()throws IOException,SQLException. |
Difference between final, finally and finalize
There are many differences between final, finally and finalize. A list of differences between final, finally and finalize are given below:No. | final | finally | finalize |
---|---|---|---|
1) | Final is used to apply restrictions on class, method and variable. Final class can't be inherited, final method can't be overridden and final variable value can't be changed. | Finally is used to place important code, it will be executed whether exception is handled or not. | Finalize is used to perform clean up processing just before object is garbage collected. |
2) | Final is a keyword. | Finally is a block. | Finalize is a method. |
==============================================================
HACKER RANK PROBLEMS:
---------------------------------------------------------------------------------------------------------------
PRG-1)
=================================================
PRG-2
No comments:
Post a Comment
Note: Only a member of this blog may post a comment.