The java.lang.String class implements Serializable, Comparable and CharSequence interfaces.
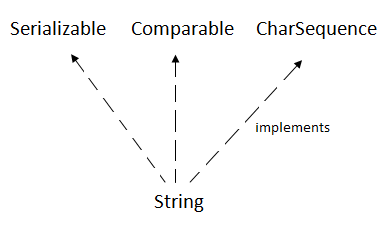
CharSequence Interface
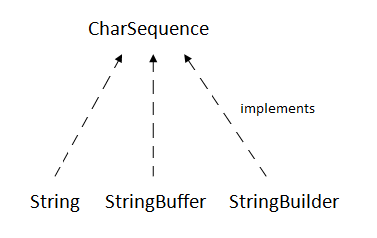
Creating a String
- String literal
- Using new keyword
String literal:
String s = "RameshQaPlatform";
Each time you create a string literal, the JVM checks the "string constant pool" first. If the string already exists in the pool, a reference to the pooled instance is returned. If the string doesn't exist in the pool, a new string instance is created and placed in
String s1 = "Welcome"; String s2 = "Welcome";
Note: String objects are stored in a special memory area known as the "string constant pool".
Why Java uses the concept of String literal?
To make Java more memory efficient (because no new objects are created if it exists already in the string constant pool).By new keyword:String strObject = new String("Java");In such case, JVM will create a new string object in normal (non-pool) heap memory, and the literal "Welcome" will be placed in the string constant pool. The variable strObject will refer to the object in a heap (non-pool).What is String literal and String Pool
Since String is one of the most used type in any application, Java designer took a step further to optimize uses of this class. They know that Strings will not going to be cheap, and that's why they come up with an idea to cache all String instances created inside double quotes e.g. "Java". These double quoted literal is known as String literal and the cache which stored these String instances are known as as String pool. In earlier version of Java, I think up-to Java 1.6 String pool is located in permgen area of heap, but in Java 1.7 updates its moved to main heap area. Earlier since it was in PermGen space, it was always a risk to create too many String object, because its a very limited space, default size 64 MB and used to store class metadata e.g. .class files. Creating too many String literals can cause java.lang.OutOfMemory: permgen space. Now because String pool is moved to a much larger memory space, it's much more safe. By the way, don't misuse memory here, always try to minimize temporary String object e.g. "a", "b" and then "ab". Always use StringBuilder to deal with temporary String object. What is string constant pool? The string constant pool, often simply referred to as the "string pool," is a special area in memory where string literals are stored. It's a feature in many programming languages, including Java, C#, and Python, that optimizes memory usage by reusing identical string literals instead of creating new instances for each occurrence of the same string.Here's how the string constant pool works:
String Literal Creation: When you create a string literal in your code (e.g.,
"hello"
), the programming language's compiler or interpreter checks if an identical string already exists in the string pool.String Reuse: If a matching string literal is found in the pool, the new string literal refers to the existing one rather than creating a new instance. This is possible because strings are immutable; they cannot be modified after creation.
Memory Efficiency: By reusing string literals, the string pool conserves memory, especially in situations where the same string literal is used multiple times throughout the program.
For example, consider the following Java code:
String str1 = "hello"; String str2 = "hello";
In this code,String pooling offers benefits in terms of memory efficiency, performance, and even certain optimizations, such as faster string comparison (since you can compare string references rather than their content). However, it's important to note that not all strings are automatically pooled; typically, only string literals are pooled by default. Strings created using thestr1
andstr2
both refer to the same string literal"hello"
in the string pool. Instead of creating two separate string objects, the Java runtime optimizes memory usage by reusing the existing string literal.new
keyword or by concatenation are not automatically part of the string pool. Some languages also offer mechanisms (e.g.,String.intern()
in Java) to explicitly add strings to the pool.Difference between String literal and String object
At high level both are String object, but main difference comes from the point that new() operator always creates a new String object. Also when you create String using literal they are interned. This will be much more clear when you compare two String objects created using String literal and new operator, as shown in below example :String a = "Java"; String b = "Java"; System.out.println(a == b); // TrueHere two different objects are created and they have different references:String c = new String("Java"); String d = new String("Java"); System.out.println(c == d); // FalseSimilarly when you compare a String literal with an String object created using new() operator using == operator, it will return false, as shown below :String e = "JDK"; String f = new String("JDK"); System.out.println(e == f); // FalseIn general you should use the string literal notation when possible. It is easier to read and it gives the compiler a chance to optimize your code. By the way any answer to this question is incomplete until you explain what is String interning, so let's see that in next section.
String interning using intern() method
Java by default doesn't put all String object into String pool, instead they gives you flexibility to explicitly store any arbitrary object in String pool. You can put any object to String pool by calling intern() method of java.lang.String class. Though, when you create using String literal notation of Java, it automatically call intern() method to put that object into String pool, provided it was not present in the pool already. This is another difference between string literal and new string, because in case of new, interning doesn't happen automatically, until you call intern() method on that object. Also don't forget to use StringBuffer and StringBuilder for string concatenation, they will reduce numberHere's a Java example that demonstrates string interning:String str1 = "hello"; String str2 = new String("hello").intern(); System.out.println(str1 == str2); // true In this example,str1
andstr2
both refer to the same string literal in the string pool becausestr2
was explicitly interned using theintern()
method.Always remember that literal Strings are returned from string pool and Java put them in pool if not stored already. This difference is most obvious, when you compare two String objects using equality operator (==). That's why it's suggested as always compare two String object using equals() method and never compare them using == operator, because you never know which one is coming from pool and which one is created using new() operator.What are the differences betweenequals()
and==
in Java?Answer: In Java,equals()
is a method used to compare the content or values of two objects, while==
is used to compare the references or memory addresses of two objects. Here's an example:
How does garbage collection work in Java?Answer:Garbage collection in Java automatically reclaims memory that is no longer in use by the program. It works by identifying objects that are no longer reachable through references and freeing up the memory occupied by those objects. The Java Virtual Machine (JVM) has a built-in garbage collector that runs periodically, determining which objects are no longer needed and reclaiming their memory.
Why strings are immutable?
Strings are immutable in many programming languages, including Java, C#, Python, and others, due to several important reasons:1)Efficiency and Performance: Immutable strings are more memory-efficient and faster in certain operations. When a string is created, it can be stored in a memory pool, and subsequent strings with the same value can reference the same memory location, reducing memory usage. Additionally, because strings are immutable, they can be cached and shared among multiple threads safely, improving performance.2)Thread Safety: Immutable strings are inherently thread-safe. In a multi-threaded environment, if one thread tries to modify a string, it cannot change the original string's content. This immutability simplifies concurrent programming and eliminates the need for explicit synchronization when working with strings.3)Security: Immutable strings enhance security. For example, when strings are used to store sensitive information like passwords or security tokens, their immutability ensures that once set, their values cannot be changed accidentally or maliciously.4)Hashing and Caching: Immutable strings are suitable for use as keys in hash tables (e.g., dictionaries) because their hash codes remain constant. If a string were mutable, and its value changed after being used as a key, it could lead to inconsistencies in data retrieval.5)Consistency: Immutability ensures that a string's value remains consistent throughout its lifetime. This predictability is crucial in maintaining program correctness and making debugging easier.6)Functional Programming: In functional programming paradigms, immutability is a fundamental concept. Immutable strings align well with functional programming principles, allowing for more predictable and maintainable code.7)String Pooling: Many programming languages implement string pooling, where common string literals are interned or shared to reduce memory usage. Immutability makes this pooling mechanism more effective because identical string literals can safely reference the same memory location8)Optimizations: Immutable strings allow compilers and runtime environments to perform various optimizations. For instance, they can avoid redundant string copying operations, leading to better performance.While immutability offers several advantages, it's essential to note that there may be situations where mutable strings are more suitable, such as when you need to frequently modify the content of a string in-place for performance reasons. In such cases, mutable string-like data structures, likeStringBuilder
in Java, can be used. However, these mutable alternatives come with trade-offs in terms of thread safety and memory management.
String Methods:
1)int length(): Returns the number of characters in the String.
"Qaplatform".length(); // returns 102)Char charAt(int i): Returns the character at ith index.
"QAPlatform".charAt(3); // returns ‘l’
3)String substring (int i): Return the substring from the ith index character to end."QAPlatform".substring(3); // returns “latform”4)String substring (int i, int j): Returns the substring from i to j-1 index."QAPlatform".substring(2, 5); // returns “Pla”5)String concat( String str): Concatenates specified string to the end of this string.String s1 = ”QaAutomation”;String s2 = ”Platform”;String output = s1.concat(s2); // returns “QaAutomationPlatform”6)int indexOf (String s): Returns the index within the string of the first occurrence of the specified string.String s = ”Learn Share Learn”;7)int indexOf (String s, int i): Returns the index within the string of the first occurrence of the specified string, starting at the specified index.String s = ”Learn Share Learn”;int output = s.indexOf("ea",3);// returns 138)Int lastIndexOf( String s): Returns the index within the string of the last occurrence of the specified string.String s = ”Learn Share Learn”;int output = s.lastIndexOf("a"); // returns 149)boolean equals( Object otherObj): Compares this string to the specified object.Boolean out = “platform”.equals(“platform”); // returns trueBoolean out = “Platform”.equals(“platform”); // returns false10)boolean equalsIgnoreCase (String anotherString): Compares string to another string, ignoring case considerations.Boolean out= “Platform”.equalsIgnoreCase(“Platform”); // returns trueBoolean out = “QaPlatform”.equalsIgnoreCase(“qaplatform”); // returns true11) int compareTo( String anotherString): Compares two string lexicographically.int out = s1.compareTo(s2); // where s1 ans s2 are// strings to be comparedThis returns difference s1-s2. If :out < 0 // s1 comes before s2out = 0 // s1 and s2 are equal.out > 0 // s1 comes after s2.12)int compareToIgnoreCase( String anotherString): Compares two string lexicographically, ignoring case considerations.int out = s1.compareToIgnoreCase(s2);// where s1 ans s2 are// strings to be comparedThis returns difference s1-s2. If :out < 0 // s1 comes before s2out = 0 // s1 and s2 are equal.out > 0 // s1 comes after s2.Note- In this case, it will not consider case of a letter (it will ignore whether it is uppercase or lowercase).13)String toLowerCase(): Converts all the characters in the String to lower case.String word1 = “HeLLo”;String word3 = word1.toLowerCase(); // returns “hello"14)String toUpperCase(): Converts all the characters in the String to upper case.String word1 = “HeLLo”;String word2 = word1.toUpperCase(); // returns “HELLO”15)String trim(): Returns the copy of the String, by removing whitespaces at both ends. It does not affect whitespaces in the middle.String word1 = “ Learn Share Learn “;String word2 = word1.trim(); // returns “Learn Share Learn”16)String replace (char oldChar, char newChar): Returns new string by replacing all occurrences of oldChar with newChar.String s1 = “javaselenium“;String s2 = s1.replace(‘a’ ,’@’); // returns “j@v@selenium”This replace method in String takes one character and replaces all of its occurrence in providedString with new character provided to it. Here is an String replace Example of changing characterString replaceSample = "This String replace Example shows how to replace one char from String";String newString = replaceSample.replace('r', 't');Output: This Stting teplace Example shows how to teplace one chat ftom SttingYou can see all occurrence of "r" has been replaced by "t".Important points:1. This replace method of String will return a new String object if there is a replace.2. It will return same String objects if there is no matching char and there is no replace.3. Replacement of String is Case Sensitive, so replacing "c" will only replace small case not Capital Case.2. Replace method to replace character sequence in Stringreplace(CharSequence target, CharSequence replacement)This String replace method replaces one character sequence with other. This method has added from JDK 1.5 onwards. Here is a String replace example of replacing character sequence form StringString replaceSample = "String replace Example of replacing Character Sequence";String newString = replaceSample.replace("re", "RE");Output: String REplace Example of REplacing Character SequenceIn this String replace Example, we have replaced "re" with "RE".Important points:1) This String replace method will throw NullPointerException if either oldCharSequence or newCharSequence is null.2) Replacement of String starts from beginning and proceed towards end. So in a String "ccc" replacing "cc" with "d" will result in "dc" rather than "cd".3. Replace method to replace all matched pattern in StringreplaceAll(String regex, String replacement)Good thing about replace method of String Class in Java is that it supports regular expression. With regex capability you can perform sophisticated Search on String and than replace characters. This String replace method replaces each matched substring with the replacement String provided. Let's see String replace example with regular expression:String replaceSample = "String replace Example with regular expression";String newString = replaceSample.replaceAll("^S","R");Output: Rtring replace Example with regular expressionThis String replace replaces any "S" wiht "R" if it comes at beginning of line "^" denotes beginning of line you can also apply pattern matching wildcards and charset while replacing String in Java.Important points:1. This String replace method will throw PatternSyntaxException in case regular expression's syntax is not valid.4. Replace method to replace first matched pattern in SStringreplaceFirst(String regex, String replacement)This String replace method is similar to above method but it only replaces first occurrence of matching pattern instead of all occurrence. Very handy some time. Here is an example of String replace with replaceFirst() method.String replaceSample = "String replace Example with replaceFirst";String newString = replaceSample.replaceFirst("re","RE");Output: String REplace Example with replaceFirstYou can see only first occurrence of "re" is replaced with "RE" while second occurrence remains same
17)boolean contains(CharSequence s):returns true or false after matching the sequence of char value.18)boolean isEmpty():checks if string is empty.
isEmpty()
:
This method checks if the string is empty, meaning it has a length of 0.
It returns true
if the string is empty, false
otherwise.
Example:
String str = "";
System.out.println(str.isEmpty()); // Output: true
String str2 = "Hello";
System.out.println(str2.isEmpty()); // Output: false
isBlank()
:
This method checks if the string is empty or consists only of whitespace characters (e.g., spaces, tabs, line breaks).
It returns true
if the string is empty or contains only whitespace, false
otherwise.
Example:
String str = " ";
System.out.println(str.isBlank()); // Output: true
String str2 = "Hello";
System.out.println(str2.isBlank()); // Output: false
- In summary,
isEmpty()
checks if the string has a length of 0, while isBlank()
checks if the string is empty or contains only whitespace characters. Use isEmpty()
when you want to check for an empty string specifically, and use isBlank()
when you want to check for a string that is empty or consists only of whitespace characters.
String s8 = "null";
String s9 = null;
System.out.println(s8.isBlank());
System.out.println(s8.isEmpty());For s8
, which is "null"
, the following will be the output:
s8.isBlank()
will return false
. This is because although "null"
contains characters, it is not considered blank because it contains non-whitespace characters.s8.isEmpty()
will return false
. This is because "null"
has a length of 4, so it is not considered empty.
For s9
, which is null
(not the string "null" but a null reference):
- If you try to call
isBlank()
or isEmpty()
on s9
, you will get a NullPointerException
because you cannot call methods on a null reference.
If you want to check if a string is "null"
(the string literal), you can compare it directly like this:
if ("null".equals(s8)) {
// s8 is the string "null"
}
Or if you want to check if a string is null (not the string literal "null"), you can do this:if (s9 == null) {
// s9 is null
}
- public static String valueOf(boolean b)
- public static String valueOf(char c)
- public static String valueOf(char[] c)
- public static String valueOf(int i)
- public static String valueOf(long l)
- public static String valueOf(float f)
- public static String valueOf(double d)
- public static String valueOf(Object o)
Java StringBuffer class:
Constructor | Description |
---|---|
StringBuffer() | creates an empty string buffer with the initial capacity of 16. |
StringBuffer(String str) | creates a string buffer with the specified string. |
StringBuffer(int capacity) | creates an empty string buffer with the specified capacity as length. |
Modifier and Type | Method | Description |
---|---|---|
public synchronized StringBuffer | append(String s) | is used to append the specified string with this string. The append() method is overloaded like append(char), append(boolean), append(int), append(float), append(double) etc. |
public synchronized StringBuffer | insert(int offset, String s) | is used to insert the specified string with this string at the specified position. The insert() method is overloaded like insert(int, char), insert(int, boolean), insert(int, int), insert(int, float), insert(int, double) etc. |
public synchronized StringBuffer | replace(int startIndex, int endIndex, String str) | is used to replace the string from specified startIndex and endIndex. |
public synchronized StringBuffer | delete(int startIndex, int endIndex) | is used to delete the string from specified startIndex and endIndex. |
public synchronized StringBuffer | reverse() | is used to reverse the string. |
public int | capacity() | is used to return the current capacity. |
public void | ensureCapacity(int minimumCapacity) | is used to ensure the capacity at least equal to the given minimum. |
public char | charAt(int index) | is used to return the character at the specified position. |
public int | length() | is used to return the length of the string i.e. total number of characters. |
public String | substring(int beginIndex) | is used to return the substring from the specified beginIndex. |
public String | substring(int beginIndex, int endIndex) | is used to return the substring from the specified beginIndex and endIndex. |
===============================================
Difference between String and StringBuffer:
No. | String | StringBuffer |
---|---|---|
1) | String class is immutable. | StringBuffer class is mutable. |
2) | String is slow and consumes more memory when you concat too many strings because every time it creates new instance. | StringBuffer is fast and consumes less memory when you cancat strings. |
3) | String class overrides the equals() method of Object class. So you can compare the contents of two strings by equals() method. | StringBuffer class doesn't override the equals() method of Object class. |
Difference between StringBuilder and StringBuffer:
No. StringBuffer StringBuilder 1) StringBuffer is synchronized i.e. thread safe. It means two threads can't call the methods of StringBuffer simultaneously. StringBuilder is non-synchronized i.e. not thread safe. It means two threads can call the methods of StringBuilder simultaneously. 2) StringBuffer is less efficient than StringBuilder. StringBuilder is more efficient than StringBuffer. 3) StringBuffer was introduced in Java 1.0 StringBuilder was introduced in Java 1.5
String StringBuffer StringBuilder
--------------------------------------------------------------------------------------------------------------
Storage Area | Constant String Pool Heap Heap
Modifiable | No (immutable) Yes( mutable ) Yes( mutable )
Thread Safe | Yes Yes No
Performance | Slow Slow but faster Fast
than String
--------------------------------------------------------------------------------------------------------------
What is mutable string
5 Reasons of Why String is final or Immutable in Java

1. String Pool
Java designer knows that String is going to be most used data type in all kind of Java applications and that's why they wanted to optimize from start. One of key step on that direction was idea of storing String literals in the String pool. The goal was to reduce temporary String object by sharing them and in order to share, they must have to be from Immutable class. You can not share a mutable object with two parties that are unknown to each other. Let's take a hypothetical example, where two reference variable is pointing to same String object:
String s1 = "Java";
String s2 = "Java";
Now if s1 changes the object from "Java" to "C++", the reference variable also got value s2="C++", which it doesn't even know about it. By making String immutable, this sharing of String literal was possible. In short, the key idea of the String pool can not be implemented without making String final or Immutable in Java.
2. Security
Java has a clear goal in terms of providing a secure environment at every level of service and String is critical in that whole security stuff. The string has been widely used as a parameter for many java classes, like for opening network connection, you can pass host and port as String, for reading files in Java you can pass the path of files and directory as String, and for opening database connection, you can pass database URL as String.
3. Use of String in Class Loading Mechanism
Another reason for making String final or Immutable was driven by the fact that it was heavily used in the class loading mechanism. As String been not Immutable, an attacker can take advantage of this fact and a request to load standard Java classes e.g. java.io.Reader can be changed to malicious class com.unknown.DataStolenReader. By keeping String final and immutable, we can at least be sure that JVM is loading the correct classes.
4. Multithreading Benefits
Since Concurrency and Multi-threading was Java's key offering, it made lot of sense to think about the thread-safety of String objects. Since it was expected that String will be used widely, making it Immutable means no external synchronization, which means much cleaner code involving sharing of String between multiple threads.
5. Optimization and Performance
Now when you make a class Immutable, you know in advance that, this class is not going to change once created. This guarantees open path for many performance optimization e.g. caching. String itself knows that I am not going to change, so String cache its hashcode. It even calculate hashcode lazily and once created, just cache it.
Pros and Cons of String being Immutable or Final in Java
Apart from the above benefits, there is one more advantage that you can count on due to String being final in Java. It's one of the most popular objects to be used as a key in hash-based collections e.g. HashMap and Hashtable. Though immutability is not an absolute requirement for HashMap keys, its much safer to use Immutable object as key than mutable ones, because if the state of a mutable object is changed during its stay inside HashMap, it would be impossible to retrieve it back, given its equals() and hashCode() method depends upon the changed attribute.Despite all these advantages, Immutability also has some disadvantages, e.g. it doesn't come without cost. Since String is immutable, it generates lots of temporary use and throw object, which creates pressure for the Garbage collector. Java designer has already thought about it and storing String literals in pool is their solution to reduce String garbage.
calculateLength
method takes a string as input. If the string is empty, it returns 0 (base case). Otherwise, it adds 1 to the length and recursively calls itself with the substring excluding the first character. This process continues until the base case is reached.
No comments:
Post a Comment
Note: Only a member of this blog may post a comment.