if
if-else
nested if -else
switch
while
do -while
for loop
for each
break
continue
array
If statement in Java is a simple decision-making statement. It is used to decide whether the statement or block of statements will be executed or not. Block of statements will be executed if the given condition is true otherwise the block of the statement will be skipped.
Here, the condition will be always in boolean form. It will be either true or false. if statement accepts only boolean value in condition. If a user doesn’t provide curly braces (‘{‘ ‘}’ ) then by default only one statement will be considered inside the if block.
Flowchart of if statement
What type of condition can have an if statement in Java?
The condition of if statement can be an expression or any boolean variable.
First, we will understand it with an expression that will return a boolean value. We can use any expression as a condition that gives results in boolean.
Example of expression:
5 > 3, It returns true because 5 is greater than 3.
5 > (3+3), It returns false because 5 is less than 6.
int a = 10;
int b = 15;
int c = 12;
a > b, It returns false because a(10) is less than b(15).
(a+b) > c, It returns true because a+b(25) is greater than c(12).
Let’s create an example, In which if the condition can take the expression of different types.
Output: The Number 5 greater than 3
The value of b is greater than a
The value of a+b is greater than c
We can directly use a boolean value as a condition in the if statement. Let’s create an example with a boolean value.
Example of expression:
true,
false
Output: The statement executes because the value is true.
The statement executes because the value is inverting of false.
logical operators:
== && || AND OR
Example:
int a=50, b=40;
if(a>b){
System.out.println("a is big");
}
example 2)
if(a<b){
System.out.println("a is smaller than b");
}
if condition is false if block of code wont execute.
2)if-else:In if statement the block of statements will be executed if the given condition is true otherwise block of the statement will be skipped. But we also want to execute some other block of code if the condition is false. In this type of scenario, we can use the if else statement in Java. There are two blocks one is if block and another is else block. If a certain condition is true, then if block will be executed otherwise else block will be executed.
syntax:
In Java, if statement having an optional block and this block is known as else block. You must think why we are saying else block is optional? Because if block can be used without else block but else block exists only with if block. The statements inside of else block will be executed only if the test expression is evaluated to false. There are a number of the situation when we want to execute the code based different situation. Suppose you want to perform TASK1 when code is satisfying the if condition otherwise you want to perform a different tasks.
RULE: You can place any code in between closing braces of if (‘}’) and else.
Flowchart of if-else statement
Example:int a=20, b=30;
if(a>b){
System.out.println("a is big");
}else{
System.out.println("b is big");
}
-------------------------------
if(a<b){
System.out.println("b is big");
}else{
System.out.println("a is big");
}
=========================================
int a=40,b=30,c=100;
if(a>10||b>12){
System.out.println("a value is-->"+a);
}else{
System.out.println("c value is-->"+c);
}
-------------------------------------------
if(a<10&&b>12){
System.out.println("a value is-->"+a);
}else{
System.out.println("c value is-->"+c);
}
==============================================
nested if else condition:
we will discuss the if else if ladder and also see the flowchart of it. In if else statement, the if block of statements will be executed if the given condition is true otherwise else block of the statement, will be executed. In if else statement the block of statements will be executed if the given condition is true otherwise block of the statement will be skipped. If we want to execute the different codes based on different conditions then we can use if-else-if. It is also known as if else if ladder statement in java. This statement is always be executed from the top down. During the execution of conditions if any condition founds true, then the statement associated with that if it is executed, and the rest of the code will be skipped. If none of the conditions is true, then the final else statement will be executed.
Flowchart of if else if ladder statement in java
syntax:
if(condition1){
condition1 statements;
}else if(condition2){
condition2 statements;
}else if(condition3){
condition3 statement;
}else if(condition4){
condition4 statements;
}
....
.}else{
false statements;
}
example:
findout in a,b,c which one is big
if(a>b&&a>c){
System.out.println("a is big");
}else if(b>c&&b>a){
System.out.println("b is big");
}else if(c>a&&c>b){
System.out.println("c is big");
}else{
System.out.println("invalid data");
}
======================================
The following program, IfElseDemo
, assigns a grade based on the value of a test score: an A for a score of 90% or above, a B for a score of 80% or above, and so on.
Input: Take marks obtained (out of 100) in each subject.
Calculate Total Marks: Sum up the marks obtained in all subjects.
Calculate Average Percentage: Divide the total marks by the total number of subjects to get the
average percentage.
Grade Calculation: Assign grades based on the average percentage achieved.
Display Results: Show the total marks, average percentage, and the corresponding grade to the user
import java.util.Scanner; // Missing import statement
public class GradeCalculator { // `Public` should be `public`
public static void main(String[] args) {
// Create a Scanner object to read input
Scanner scanner = new Scanner(System.in);
// Step 1: Input marks
System.out.print("Enter the number of subjects: ");
int totalSubjects = scanner.nextInt(); // Variable name fixed (should match usage below)
int[] marks = new int[totalSubjects];
int totalMarks = 0;
// Get marks for each subject
for (int i = 0; i < totalSubjects; i++) { // `totalSubject` was misspelled
System.out.print("Enter marks for subject " + (i + 1) + ": ");
marks[i] = scanner.nextInt();
totalMarks += marks[i]; // Add the marks to totalMarks
}
// Step 2: Calculate Average Percentage
double averagePercentage = (double) totalMarks / totalSubjects; // Fixed incorrect formula
// Step 3: Grade Calculation
char grade;
if (averagePercentage >= 90) {
grade = 'A';
} else if (averagePercentage >= 80) {
grade = 'B';
} else if (averagePercentage >= 70) { // Missing parenthesis `()`
grade = 'C'; // Missing semicolon `;`
} else if (averagePercentage >= 60) { // Missing parenthesis `()`
grade = 'D'; // Missing semicolon `;`
} else {
grade = 'F'; // Fixed duplicate 'D' grade
}
// Step 4: Display the results
System.out.println("\nTotal Marks: " + totalMarks); // `println` should be lowercase
System.out.println("Average Percentage: " + String.format("%.2f", averagePercentage) + "%"); // Fixed extra backslash
System.out.println("Grade: " + grade);
// Close the scanner
scanner.close();
}
}
=======================================================
Exercise:on Nested if else
Instructions
Write a program that interprets the Body Mass Index (BMI) based on a user's weight and height.
It should tell them the interpretation of their BMI based on the BMI value.
Under 18.5 they are underweight
Over 18.5 but below 25 they have a normal weight
Over 25 but below 30 they are slightly overweight
Over 30 but below 35 they are obese
Above 35 they are clinically obese.
The BMI is calculated by dividing a person's weight (in kg) by the square of their height (in m):
Warning you should round the result to the nearest whole number. The interpretation message needs to include the words in bold from the interpretations above. e.g. underweight, normal weight, overweight, obese, clinically obese.
Example Input
weight = 85
height = 1.75
Example Output
85 ÷ (1.75 x 1.75) = 27.755102040816325
Your BMI is 28, you are slightly overweight.
The testing code will check for print output that is formatted like one of the lines below:
"Your BMI is 18, you are underweight."
"Your BMI is 22, you have a normal weight."
"Your BMI is 28, you are slightly overweight."
"Your BMI is 33, you are obese."
"Your BMI is 40, you are clinically obese."
========================================================================
EXERCISE:
This is a Difficult Challenge 💪
Instructions
Write a program that works out whether if a given year is a leap year. A normal year has 365 days, leap years have 366, with an extra day in February. The reason why we have leap years is really fascinating, this video does it more justice:
https://www.youtube.com/watch?v=xX96xng7sAE
This is how you work out whether if a particular year is a leap year.
on every year that is evenly divisible by 4
**except** every year that is evenly divisible by 100
**unless** the year is also evenly divisible by 400
e.g. The year 2000:
2000 ÷ 4 = 500 (Leap)
2000 ÷ 100 = 20 (Not Leap)
2000 ÷ 400 = 5 (Leap!)
So the year 2000 is a leap year.
But the year 2100 is not a leap year because:
2100 ÷ 4 = 525 (Leap)
2100 ÷ 100 = 21 (Not Leap)
2100 ÷ 400 = 5.25 (Not Leap)
Warning your output should match the Example Output format exactly, even the positions of the commas and full stops.
Example Input 1
2400
Example Output 1
Leap year.
Example Input 2
1989
Example Output 2
Not leap year.
e.g. When you hit run, this is what should happen:
Hint
Try to visualise the rules by creating a flow chart on www.draw.io
If you really get stuck, you can see the flow chart I created:
https://bit.ly/36BjS2D
===========================================================================
Exercise:write a program for below scenario
print "Welcome to the Rollercoaster" and take a height parameter in cm to measure rider is eligible to ride or not.
If height >= 120 then print "You can ride the rollercoaster" else print "Sorry, you have to grow taller before you can ride." and take one age parameter to pay the ticket bill.
if age <12 then pay the ticket bill :$5 and age is <=18 then pay the ticket bill $7 and age is > 18 then pay the bill $12
and take a want a photo boolean type parameter with Y or N if you want to take a photo then you need to pay extra $3 for the ticket else pay the ticket bill without photo amount
Flow Diagram:
==========================================================
EXERCISE: Write a program for below scenario
Congratulations, you've got a job at Python Pizza. Your first job is to build an automatic pizza order program.
Based on a user's order, work out their final bill.
Small Pizza: $15
Medium Pizza: $20
Large Pizza: $25
Pepperoni for Small Pizza: +$2
Pepperoni for Medium or Large Pizza: +$3
Extra cheese for any size pizza: + $1
Example Input
size = "L"
add_pepperoni = "Y"
extra_cheese = "N"
Example Output
Your final bill is: $28.
💪 This is a Difficult Challenge 💪
Instructions
You are going to write a program that tests the compatibility between two people.
To work out the love score between two people:
Take both people's names and check for the number of times the letters in the word TRUE occurs.
Then check for the number of times the letters in the word LOVE occurs.
Then combine these numbers to make a 2 digit number.
For Love Scores less than 10 or greater than 90, the message should be:
"Your score is **x**, you go together like coke and mentos."
For Love Scores between 40 and 50, the message should be:
"Your score is **y**, you are alright together."
Otherwise, the message will just be their score. e.g.:
"Your score is **z**."
e.g.
name1 = "Angela Yu"
name2 = "Jack Bauer"
T occurs 0 times
R occurs 1 time
U occurs 2 times
E occurs 2 times
Total = 5
L occurs 1 time
O occurs 0 times
V occurs 0 times
E occurs 2 times
Total = 3
Love Score = 53
Print: "Your score is 53."
Example Input 1
name1 = "Kanye West"
name2 = "Kim Kardashian"
Example Output 1
Your score is 42, you are alright together.
Example Input 2
name1 = "Brad Pitt"
name2 = "Jennifer Aniston"
Example Output 2
Your score is 73.
The testing code will check for print output that is formatted like one of the lines below:
"Your score is 47, you are alright together."
"Your score is 125, you go together like coke and mentos."
"Your score is 54."
Score Comparison
Not sure you're getting the correct score for the exercise? Use this table to check your code's score against mine.
Name 1 | Name 2 | Score |
Catherine Zeta-Jones | Michael Douglas | 99 |
Brad Pitt | Jennifer Aniston | 73 |
Prince William | Kate Middleton | 67 |
Angela Yu | Jack Bauer | 53 |
Kanye West | Kim Kardashian | 42 |
Beyonce | Jay-Z | 23 |
John Lennon | Yoko Ono | 18 |
switch:The switch statement Java is like the if-else-if ladder statement. To reduce the code complexity of the if-else-if ladder switch statement comes. Switch statement is the alternate the of if else if ladder statement.
The switch statement executes one block of the statement from multiple blocks of statements based on condition. In the switch statements, we have a number of choices and we can perform a different task for each choice.
Rules of the Switch statement Java
- An expression can be of byte, short, int, long, enum types, String and some wrapper types like Byte, Short, Int, and Long. You can place a variable or an expression in between brackets of switch().
- You can create any number of cases in switch statement.
- The case value must be literal or constant. The case value type should be a type of expression.
- Each case should be unique. If you create duplicate case value, it will throw compile-time error.
- Each case has a break statement which is optional. It is used inside the switch to terminate a statement sequence and jumps the control after the switch expression.
- If a break statement is not used in any case the execution will continue into the next case until a break statement is reached.
- In the switch, we can have an optional default case that must be placed at the end of the switch statement. If none of the cases are true, then this default case will be executed.The default case doesn’t need break statement.
example:
findingout entered character is vowel or consonent
public class SwitchDemo{
public static void findCharVowelOrConsonent(char c){
switch(c){
case 'a':
System.out.println("entered character is a vowel:"+c);
break;
case 'e':
System.out.println("entered character is a vowel:"+c);
break;
case 'i':
System.out.println("entered character is a vowel:"+c);
break;
case 'o':
System.out.println("entered character is a vowel:"+c);
break;
case 'u'
System.out.println("entered character is a vowel:"+c);
break;
default:
System.out.println("entered character is not a vowel:"+c);
} //switch ending
} //class ending
==================================
Example: Program to make a calculator using switch case in Java
import java.util.Scanner;
public class JavaExample {
public static void calculate(char operator) {
double num1, num2;
//creating an object for the Scanner class.
Scanner sc = new Scanner(System.in);
System.out.print("Enter first number:");
num1 = sc.nextDouble();
System.out.print("Enter second number:");
num2 = sc.nextDouble();
sc.close();
double output;
switch(operator)
{
case '+':
output = num1 + num2;
break;
case '-':
output = num1 - num2;
break;
case '*':
output = num1 * num2;
break;
case '/':
output = num1 / num2;
break;
default:
System.out.printf("You have entered wrong operator");
}
System.out.println(num1+" "+operator+" "+num2+": "+output);
}
}
class SwitchDemo2 { public static void main(String[] args) { int month = 2; int year = 2000; int numDays = 0; switch (month) { case 1: case 3: case 5: case 7: case 8: case 10: case 12: numDays = 31; break; case 4: case 6: case 9: case 11: numDays = 30; break; case 2: if (((year % 4 == 0) && !(year % 100 == 0)) || (year % 400 == 0)) numDays = 29; else numDays = 28; break; default: System.out.println("Invalid month."); break; } System.out.println("Number of Days = " + numDays); } }
This is the output from the code:
Number of Days = 29
In Java SE 7 and later, you can use a String
object in the switch
statement's expression. The following code example, StringSwitchDemo
, displays the number of the month based on the value of the String
named month
:
public class StringSwitchDemo { public static int getMonthNumber(String month) { int monthNumber = 0; if (month == null) { return monthNumber; } switch (month.toLowerCase()) { case "january": monthNumber = 1; break; case "february": monthNumber = 2; break; case "march": monthNumber = 3; break; case "april": monthNumber = 4; break; case "may": monthNumber = 5; break; case "june": monthNumber = 6; break; case "july": monthNumber = 7; break; case "august": monthNumber = 8; break; case "september": monthNumber = 9; break; case "october": monthNumber = 10; break; case "november": monthNumber = 11; break; case "december": monthNumber = 12; break; default: monthNumber = 0; break; } return monthNumber; } public static void main(String[] args) { String month = "August"; int returnedMonthNumber = StringSwitchDemo.getMonthNumber(month); if (returnedMonthNumber == 0) { System.out.println("Invalid month"); } else { System.out.println(returnedMonthNumber); } } }
The output from this code is 8
.
The String
in the switch
expression is compared with the expressions associated with each case
label as if the String.equals
method were being used. In order for the StringSwitchDemo
example to accept any month regardless of case, month
is converted to lowercase (with the toLowerCase
method), and all the strings associated with the case
labels are in lowercase.
Note: This example checks if the expression in the switch
statement is null
. Ensure that the expression in any switch
statement is not null to prevent a NullPointerException
from being thrown.
The Incerment and Decrement Operators
/**
* This program demonstrates the ++ and -- operators.
*/
public class IncrementDecrement
{
public static void main(String[] args)
{
int number = 50;
// Display the value in number.
System.out.println("Number is " + number);
// Increment number.
number++;
// Display the value in number.
System.out.println("Now, number is " + number);
// Decrement number.
number--;
// Display the value in number.
System.out.println("Now, number is " + number);
}
}
Now, number is 51
Now, number is 50
++number;
number++
--number;
number--
a = 5;
b = 10 + (++a);
a = 5;
b = 10 + (a++);
Loops/Iterators:
Loops are used in programming to repeat a specific block of code until certain
condition is met false.
The While loop
A while loop allows code to be executed repeatedly until a condition is satisfied. If the number of iterations is not fixed, it is recommended to use a while loop. It is also known as an entry control loop. In this article, we will discuss how we can use while loop java.
Firstly, the condition will be evaluated:
- If it returns true, then the body of the while loop will be executed. In the body of while loop the statements contain an update value for the variable being processed for the next iteration.
- If it returns false, then the loop terminates which marks the end of its life cycle
initialization;
while(condition){ //<=,>=
l+statements;
increment(++)/decrement(--);
}
Note: if while condition is true enters into the while body & executes the statements;
example:
printing from 1 to 10;
int i=1;
while(i<=10){//1<=10-T/2<=10->T/3<=10-T/4<=10-T/5<=10-T/6<=10-T/7<=10-T/8<=10-T/9<=10-T/10==10-T/11<=10-F
System.out.print(i+",");
i++; //i=1+1=2/i=2+1=3/i=3+1=4/i=4+1=5/i=5+1=6/i=6+1=7/i=7+1=8/i=8+1=9/i=9+1=10/i=10+1=11
}
output:1,2,3,4,5,6,7,8,9,10,
exercise:
print the values from 10 to 1
int j=10;
while(j>=1){//10>=1-T/9>=1-T/8>=1-T/7>=1-T//6>=1-T//5>=1-T//4>=1-T//3>=1-T//2>=1-T//1>=1-T//0>=1-F
System.out.print(j+",");
j--; /10-1=9/9-1=8/8-1=7//7-1=6//6-1=5/5-1=4//4-1=3//3-1=2//2-1=1//1-1=0
}
output:10,9,8,7,6,5,4,3,2,1
---------------------------------------------------------------------
Java Infinitive while Loop: If the condition of while loop will never false. Then it’s an infinite while loop. Here, below is example of infinite while loop.
It is an infinite loop. Because this is because the condition is i > 0 which would always be true as we are incrementing the value of i inside while loop.
If we pass true in while loop condition. Then it will be an infinite loop.
================================================
int i=1;
while(i<=1){
System.out.println("java");
i++;
}
----------------------------------------------------
int i=2;
while(i<=20){
System.out.print(i+",");
i*i;
}
------------------------------------------------------------------------
public int findSum(int n){
int sum=0; //n
int i=1;
while(i<=n){ //
sum=sum+i;//
i++; //
} //while loop end
return sum;
} //find sum method end
---------------------------------------------------------------------
do-while:
It is like a while loop, It also allows code to be executed repeatedly until a condition is satisfied. The only difference that it checks for the condition after the execution of the body of the loop. It is also known as Exit Control Loop. Here we will discuss how to use do while loop in Java
Working of do while loop in Java?
First, the statements inside the loop execute and then the condition gets evaluated.
If the condition returns true then the control gets transferred to the “do” and it again executes the statement that presented in the loop body.
If the condition returns false then control flow directly it jumps to out of the loop and it executes the next statement after the loop.
NOTE: The do-while loop executes the code at least one time because it checks the condition after execution of the body.
initialization;
do{
statements;
increment/decrement;
}while(condition);
print 1 to 10 numbers:
int i=1;
do{
System.out.print(i+",");
i++; //i=2 /i=3/i=4/i=5/i=6/i=7/i=8/i=9/i=10/i=11
}while(i<=10); //1<=10-T/2<=10-T/3<=10-T/4<=10-T/5<=10-T/6<=10-T/7<=10-T/8<=10/9<=10=T/10=10-T
output:
1,2,3,4,5,6,7,8,9,10
print 10 to 1:
int i=10;
do{
System.out.print(i+",");
i--; //10-1-9//9-1=8
}while(i>=1);
output:
10,9,8,7,6,5,4,3,2,1
Infinitive do while: If the condition of loop will never false. Then it’s infinite do while loop.
It is an infinite loop. Because this is because the condition is i > 0 which would always be true as we are incrementing the value of i inside body of the loop.
Another example:
import java.util.Scanner; // needed for Scanner Class
/**
* This program demonstrate do while loop.
*/
public class AddNumbers
{
public static void main(String[] args)
{
int value; // to hold data entered by the user
int sum = 0; // initialize the sum
char choice; // to hold 'y' or 'n'
// Create a Scanner object for keyboard input.
Scanner console = new Scanner(System.in);
do
{
// Get the value from the user.
System.out.print("Enter integer: ");
value = console.nextInt();
// add value to sum
sum = sum + value;
// Get the choice from the user to add more number
System.out.print("Enter Y for yes or N for no: ");
choice = console.next().charAt(0);
}
while ((choice == 'y') || (choice == 'Y'));
//Display the total.
System.out.println("Sum of the integers: " + sum);
}
}
Enter Y for yes or N for no: y
Enter integer: 8
Enter Y for yes or N for no: y
Enter integer: 4
Enter Y for yes or N for no: n
Sum of the integers: 18
import java.util.Scanner; // needed for Scanner Class
/**
* This program implements number guessing game.
*/
public class GuessMyNumber
{
public static void main(String[] args)
{
int num; // to hold random number
int guess; // to hold the number guessed by the user
// Create a Scanner object for keyboard input.
Scanner console = new Scanner(System.in);
// Get random number between 1 to 100.
num = (int) (Math.random() * 100) + 1;
do
{
System.out.print("Enter an integer between 1 to 100: ");
guess = console.nextInt();
if (guess == num)
{
System.out.println("You guessed the correct number.");
}
else if (guess < num)
{
System.out.println(
"Your guess is lower than the number.\nGuess again!");
}
else
{
System.out.println(
"Your guess is higher than the number.\nGuess again!");
}
}
while (guess != num);
}
}
Your guess is higher than the number.
Guess again!
Enter an integer between 1 to 100: 23
Your guess is higher than the number.
Guess again!
Enter an integer between 1 to 100: 10
Your guess is lower than the number.
Guess again!
Enter an integer between 1 to 100: 21
Your guess is higher than the number.
Guess again!
Enter an integer between 1 to 100: 20
You guessed the correct number.
------------------------------------------------------------------------------
for Loop: If a user wants to execute the block of code several times. It means the number of iterations is fixed. Then you should use the for loop java. JAVA provides a concise way of writing the loop structure. It consists of four parts:
1. Initialization
2. Condition
3. Body of for loop
4. Increment/decrement
for(initialization;condition;increment/decrement){
statements;
}
1. Initialization: Initialization is the first part of the loop and it happens only one time. It marks the start point of the loop. Here, you can declare and initialize the variable, or you can use any already initialized variable.
2. Condition: Condition is the second part of the loop and we must provide/write a condition that must return boolean value either true or false. It is executed each time to test the condition of the loop. It continues execution until the condition is false.
3. Body of for loop: It contains a block of code that executes each time after evaluating the condition true.
4. Increment/decrement: It is used to update the index value of the variable.
print 1 to 10 numbers with for loop:
for(int i=1;i<=10;i++){//1<=10-T/1+1-2/2<=10-T/2+1=3/3<=10-T/3+1=4/4<=10-T/4+1=5/5<=10-T/5+1=6/6<=10-T/6+1=7/7<=10-T/7+1=8/8<=10-T/8+1=9/9<=10-T/9+1=10/10==10-T/10+1-11/11<=10-F
System.out.print(i+"\t");
}
output:1 2 3 4 5 6 7 8 9 10
Explanation: In this example, we want to print the table of 1 so we used for loop. Here, we declared a variable:
It is the initialization part of the loop. It is the start point of the loop to print the count, so we initialized it with 1.
It is the condition of for loop which will be tested/evaluate each time before execution of statements of body.
It is the body of the loop which will execute only if the condition of loop returns true.
It is an increment/decrement part in a loop. In this example, we used the unary operator to change the index value of the variable. It will increment the value of “i” each time.
NOTE: After incrementing the value of “i” compiler again test the condition of for loop.
print 10 to 1 using for loop
for(int i=10;i>=1;i--){//10>=1-T/10-1=9/9>=1-T/9-1=8/8>=1-T/8-1=7/7>=1-T/7-1=6/6>=1-T/6-1=5/5>=1-T/5-1=4/4>=1-T/4-1=3/3>=1-T/3-1=2/2>=1-T/2-1=1/1=1-T/1-1=0/0>=1-F
System.out.print(i+"\t");
}
10 9 8 7 6 5 4 3 2 1
================================
how many times below program will execute?
for(int i=2;i<=20;i*i){ //2<=20-T/2*2=4/4<=20-T/4*4=16/16<=20-T/16*16=256/256<=20-F
System.out.println(i);
}
output:2 4 16
------------------------------------------
for(int i=1;i<=10;i--){//1<=10-T/1-1=0/0<=10-T/0-1=-1/-1<=10-T
System.out.println("hello");
}
hello hello
-----------------------------------
Infinite for loop: In infinite for loop the condition always returns true.
You can see the importance of Boolean expression and increment/decrement operation co-ordination. The initialization part set the value of variable i = 0, the Boolean expression always returns true because i is incrementing by 1. This would eventually lead to the infinite loop condition.
===========================================
write an instance method to find out the sum of n numbers:
public int findSum(int n){
int sum=0;
for(int j=1;j<=n;j++){
sum=sum+j;
} //for loop ending
return sum;
} //findsum method ending
--------------------------------------------------------
import java.util.Scanner; //Needed for Scanner class
/**
* This program demonstrates a
* counter controlled for loop.
*/
public class LogTable
{
public static void main(String[] args)
{
int maxValue; // Maximum value to display
// Create a Scanner object for keyboard input.
Scanner console = new Scanner(System.in);
System.out.println("Table of sequence and their logarithms.");
// Get the maximum value to display.
System.out.print("Enter last number : ");
maxValue = console.nextInt();
for (int i = 1; i <= maxValue; i++)
{
System.out.println(i + "\t" + Math.log(i));
}
}
}
Enter last number : 6
1 0.0
2 0.6931471805599453
3 1.0986122886681098
4 1.3862943611198906
5 1.6094379124341003
6 1.791759469228055
The Nested loop:
Output:
1,1
1,2
1,3
2,1
2,2
2,3
3,1
3,2
3,3
Explanation: In this example, we have two for loop, one is an outer loop and another is an inner loop. The outer and inner loop is initialized by variable and has some condition. The inner loop iterations are totally dependent on the outer loop.
If i = 1 then inner loop are executing three times for j = 1, j = 2, j = 3, Same for the i = 2 and i = 3.
/**
* This program displays a rectangular pattern
* of stars.
*/
public class RectangularPattern
{
public static void main(String[] args)
{
final int MAXROWS = 4,
MAXCOLS = 5;
for (int i = 1; i <= MAXROWS; i++)
{
for (int j = 1; j <= MAXCOLS; j++)
{
System.out.print("*");
}
System.out.println();
}
}
}
*****
*****
*****
/**
* This program displays a right angular triangle pattern.
*/
public class TrianglePattern
{
public static void main(String[] args)
{
final int SIZE = 6;
for (int i = 1; i <= SIZE; i++)
{
for (int j = 1; j <= i; j++)
{
System.out.print("*");
}
System.out.println();
}
}
}
**
***
****
*****
******
The break and continue Statement
- break: If a break statement is encountered inside a loop, the loop is immediately terminated, and the control of the program goes to the next statement following the loop. It is used along with if statement in loops. If the condition is true, then the break statement terminates the loop immediately.
/**
* This program demonstrates
* break to exit a loop.
*/
public class BreakDemo
{
public static void main(String[] args)
{
for (int i = 1; i <= 10; i++)
{
if (i == 5)
{
break; // terminate loop if i is 5
}
System.out.print(i + " ");
}
System.out.println("Loop is over.");
}
}
/**
* This program demonstrates continue
* to skip remaining statements of iteration.
*/
public class ContinueDemo
{
public static void main(String[] args)
{
for (int i = 1; i <= 10; i++)
{
if (i % 2 == 0)
{
continue; // skip next statement if i is even
}
System.out.println(i + " ");
}
}
}
The continue
statement skips the current iteration of a for
, while
, or do-while
loop. The unlabeled form skips to the end of the innermost loop's body and evaluates the boolean
expression that controls the loop. The following program, ContinueDemo
, steps through a String
, counting the occurrences of the letter "p". If the current character is not a p, the continue
statement skips the rest of the loop and proceeds to the next character. If it is a "p", the program increments the letter count.
class ContinueDemo { public static void main(String[] args) { String searchMe = "peter piper picked a " + "peck of pickled peppers"; int max = searchMe.length(); int numPs = 0; for (int i = 0; i < max; i++) { // interested only in p's if (searchMe.charAt(i) != 'p') continue; // process p's numPs++; } System.out.println("Found " + numPs + " p's in the string."); } }
Here is the output of this program:
Found 9 p's in the string.
==============================================
for-each loop:
it is used for arrays and collections, it iterates the elements in forward direction
Syntax:
for(datatype variablename:arrayname/collectionname){
statements;
}
=============================================
Introduction to Array:
For example, if we want to store the names of 100 people then we can create an array of the string type that can store 100 names.
String[] array = new String[100];
Here, the above array cannot store more than 100 names. The number of values in a Java array is always fixed.
- Code Optimization: It makes the code optimized, we can retrieve or sort the data efficiently.
- Random access: We can get any data located at an index position.
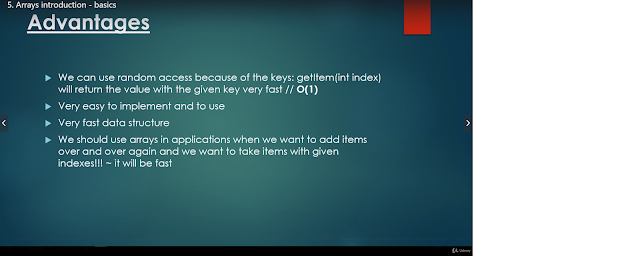
Disadvantages:
- Size Limit: We can store only the fixed size of elements in the array. It doesn't grow its size at runtime.
- To solve this problem, collection framework is used in Java which grows automatically.

Declaring Array Variables/
How to declare an array in Java?
In Java, here is how we can declare an array.
dataType[] arrayName;
- dataType - it can be primitive data types like
int
,char
,double
,byte
, etc. or Java objects - arrayName - it is an identifier
For example,
double[] data;
Here, data is an array that can hold values of type double
.
int[] list;
String[] fruits;
Rectangle[] boxes;
But, how many elements can array this hold?
Good question! To define the number of elements that an array can hold, we have to allocate memory for the array in Java. For example,
// declare an array
double[] data;
// allocate memory
data = new double[10];
Here, the array can store 10 elements. We can also say that the size or length of the array is 10.
In Java, we can declare and allocate the memory of an array in one single statement. For example,
double[] data = new double[10];
Creating Array Object
(or)
datatype arrayname[]=new datatype[size];
(or)
datatype arrayname[]=new datatype[]{e1,e2,e3,e4,e5};
Examples:
list = new int[10];
fruits = new String[5];
boxes = new Rectangle[4];
int marks = new int[5];

How to Initialize Arrays in Java?
In Java, we can initialize arrays during declaration. For example
double[] prices = {10.5, 20.1, 14, 39};
,
//declare and initialize and array
int[] age = {12, 4, 5, 2, 5};
Here, we have created an array named age and initialized it with the values inside the curly brackets.
Note that we have not provided the size of the array. In this case, the Java compiler automatically specifies the size by counting the number of elements in the array (i.e. 5).
In the Java array, each memory location is associated with a number. The number is known as an array index. We can also initialize arrays in Java, using the index number. For example,
// declare an array
int[] age = new int[5];
// initialize array
age[0] = 12;
age[1] = 4;
age[2] = 5;
..
Note:
- Array indices always start from 0. That is, the first element of an array is at index 0.
- If the size of an array is n, then the last element of the array will be at index n-1.
How to Access Elements of an Array in Java?
We can access the element of an array using the index number. Here is the syntax for accessing elements of an array,
// access array elements
array[index]
Let's see an example of accessing array elements using index numbers.
Example: Access Array Elements
class Main {
public static void main(String[] args) {
// create an array
int[] age = {12, 4, 5, 2, 5};
// access each array elements
System.out.println("Accessing Elements of Array:");
System.out.println("First Element: " + age[0]);
System.out.println("Second Element: " + age[1]);
System.out.println("Third Element: " + age[2]);
System.out.println("Fourth Element: " + age[3]);
System.out.println("Fifth Element: " + age[4]);
}
}
class Main {
public static void main(String[] args) {
// create an array
int[] age = {12, 4, 5, 2, 5};
// access each array elements
System.out.println("Accessing Elements of Array:");
System.out.println("First Element: " + age[0]);
System.out.println("Second Element: " + age[1]);
System.out.println("Third Element: " + age[2]);
System.out.println("Fourth Element: " + age[3]);
System.out.println("Fifth Element: " + age[4]);
}
}
Output
Accessing Elements of Array: First Element: 12 Second Element: 4 Third Element: 5 Fourth Element: 2 Fifth Element: 5
The following program, ArrayDemo
, creates an array of integers, puts some values in the array, and prints each value to standard output.
class ArrayDemo { public static void main(String[] args) { // declares an array of integers int[] anArray; // allocates memory for 10 integers anArray = new int[10]; // initialize first element anArray[0] = 100; // initialize second element anArray[1] = 200; // and so forth anArray[2] = 300; anArray[3] = 400; anArray[4] = 500; anArray[5] = 600; anArray[6] = 700; anArray[7] = 800; anArray[8] = 900; anArray[9] = 1000; System.out.println("Element at index 0: " + anArray[0]); System.out.println("Element at index 1: " + anArray[1]); System.out.println("Element at index 2: " + anArray[2]); System.out.println("Element at index 3: " + anArray[3]); System.out.println("Element at index 4: " + anArray[4]); System.out.println("Element at index 5: " + anArray[5]); System.out.println("Element at index 6: " + anArray[6]); System.out.println("Element at index 7: " + anArray[7]); System.out.println("Element at index 8: " + anArray[8]); System.out.println("Element at index 9: " + anArray[9]); } }
The output from this program is:
Element at index 0: 100 Element at index 1: 200 Element at index 2: 300 Element at index 3: 400 Element at index 4: 500 Element at index 5: 600 Element at index 6: 700 Element at index 7: 800 Element at index 8: 900 Element at index 9: 1000
marks[4] = 30; //stores 30 in fifth element of array.
Example 1
import java.util.Scanner; //Needed for Scanner class
/**
* This program shows values being read into an array's
* elements and then displayed.
*/
public class ArrayDemo1
{
public static void main(String[] args)
{
final int SIZE = 5; // size of array
// Create an array to hold employee salary.
double[] salaries = new double[SIZE];
// Create a Scanner object for keyboard input.
Scanner console = new Scanner(System.in);
System.out.println("Enter the salaries of " + SIZE
+ " employees.");
// Get employees' salary.
for (int i = 0; i < SIZE; i++)
{
salaries[i] = console.nextDouble();
}
// Display the values stored in the array.
System.out.println("The salaries are:");
for (int i = 0; i < SIZE; i++)
{
System.out.println(salaries[i]);
}
}
}
234
789
123
456
453
The salaries are:
234.0
789.0
123.0
456.0
453.0
1.Array length
2)how can you get the last element position of the array.
arrayname.length-1;
3)how can you get the particular position element from array.
arrayname[index]
ArrayIndexOutOfBoundsException Occurrence Scenario:
1)if index value given as negative, then system will throw ArrayIndexOutOfBoundsException
2)If we enter/insert element at wrong index in the array or more than the size of the array index inserting elements will throw ArrayIndexOutOfBoundsException
3) Array size is zero and trying to access array elements will throw ArrayIndexOutOfBoundsException
Another Example:
int[] arr=new int[5]; //declaring array
//how to insert the elements into array
arrayname[index]=value;
arr[0]=10;
arr[1]=15;
arr[2]=20;
arr[3]=25;
arr[4]=30;
arr[5]=45;
arr[10]=50; //this will throw ArrayIndexOutOfBoundsException
int arr[]=[10,15,20,25,30];
//index 0 1 2 3 4
In the above example, notice that we are using the index number to access each element of the array.
We can use loops to access all the elements of the array at once
Looping Through Array Elements
In Java, we can also loop through each element of the array. For example,
how to print an array?using for each loop
//for(datatype variablename:arrayname/collectionname){statements}
for(int a:arr){
System.out.println(a);
}
10 15 20 25 30
using normal for loop:
//for(initialization;condition;increment/decrement){statements}
for(int i=0;i<=arr.length-1;i++){//0<=4-T/0+1=1/1<=4-T/i=1+1=2/2<=4-T/i=2+1=3/3<=4-T/i=3+1=4/4==4-T/4+1=5/5<=4-F
System.out.println(arr[i]); //arr[0]/arr[1]/arr[2]/arr[3]/arr[4]
}
10,15,20,25,30
printing an array in reverse order
for(int i=arr.length-1;i>=0;i--){//i=5-1=4,/4>=0-T/4-1=3//3>=0-T/3-1=2/2>=0-T/2-1=1/1>=0-T/1-1=0//0==0-T/0-1=-1//-1>=0-F
System.out.println(arr[i]);//arr[4],arr[3],arr[2],arr[1],arr[0]
}
o/p:
30,25,20,15,10
==============================================
Example: Using For Loop
class Main {
public static void main(String[] args) {
// create an array
int[] age = {12, 4, 5};
// loop through the array
// using for loop
System.out.println("Using for Loop:");
for(int i = 0; i < age.length; i++) {
System.out.println(age[i]);
}
}
}
Output
Using for Loop: 12 4 5
In the above example, we are using the for Loop in Java to iterate through each element of the array. Notice the expression inside the loop,
age.length
Here, we are using the length
property of the array to get the size of the array.
We can also use the for-each loop to iterate through the elements of an array. For example,
Example: Using the for-each Loop
class Main {
public static void main(String[] args) {
// create an array
int[] age = {12, 4, 5};
// loop through the array
// using for loop
System.out.println("Using for-each Loop:");
for(int a : age) {
System.out.println(a);
}
}
}
Output
Using for-each Loop: 12 4 5
7.2 Processing Array Elements
/**
* This program displays even values
* of array.
*/
public class EvenArray
{
public static void main(String[] args)
{
int[] list = { 2, 5, 16, 18, 21 };
// Display all even elements of array
System.out.println("The even numbers of array:");
for (int i = 0; i < list.length; i++)
{
if (list[i] % 2 == 0)
{
System.out.print(list[i] + " ");
}
}
System.out.println();
}
}
2 16 18
4
5
Example: Compute Sum and Average of Array Elements
class Main {
public static void main(String[] args) {
int[] numbers = {2, -9, 0, 5, 12, -25, 22, 9, 8, 12};
int sum = 0;
Double average;
// access all elements using for each loop
// add each element in sum
for (int number: numbers) {
sum += number;
}
// get the total number of elements
int arrayLength = numbers.length;
// calculate the average
// convert the average from int to double
average = ((double)sum / (double)arrayLength);
System.out.println("Sum = " + sum);
System.out.println("Average = " + average);
}
}
class Main {
public static void main(String[] args) {
int[] numbers = {2, -9, 0, 5, 12, -25, 22, 9, 8, 12};
int sum = 0;
Double average;
// access all elements using for each loop
// add each element in sum
for (int number: numbers) {
sum += number;
}
// get the total number of elements
int arrayLength = numbers.length;
// calculate the average
// convert the average from int to double
average = ((double)sum / (double)arrayLength);
System.out.println("Sum = " + sum);
System.out.println("Average = " + average);
}
}
Output:
Sum = 36 Average = 3.6
In the above example, we have created an array of named numbers. We have used the for...each
loop to access each element of the array.
Inside the loop, we are calculating the sum of each element. Notice the line,
int arrayLength = number.length;
Here, we are using the length attribute of the array to calculate the size of the array. We then calculate the average using:
average = ((double)sum / (double)arrayLength);
As you can see, we are converting the int
value into double
. This is called type casting in Java.
Copying arrays

If want to allocate a new array and copy elements from one to the other.
double[] list1 = {3.9, 6.7, 13.3};
double[] list2 = new double [list1.length];
for (int i = 0; i < list1.length; i++)
{
list2[i] = list1[i];
}
In Java, we can copy one array into another. There are several techniques you can use to copy arrays in Java.
1. Copying Arrays Using Assignment Operator
Let's take an example,
class Main {
public static void main(String[] args) {
int [] numbers = {1, 2, 3, 4, 5, 6};
int [] positiveNumbers = numbers; // copying arrays
for (int number: positiveNumbers) {
System.out.print(number + ", ");
}
}
}
Output:
1, 2, 3, 4, 5, 6
In the above example, we have used the assignment operator (=
) to copy an array named numbers to another array named positiveNumbers.
This technique is the easiest one and it works as well. However, there is a problem with this technique. If we change elements of one array, corresponding elements of the other arrays also change. For example,
class Main {
public static void main(String[] args) {
int [] numbers = {1, 2, 3, 4, 5, 6};
int [] positiveNumbers = numbers; // copying arrays
// change value of first array
numbers[0] = -1;
// printing the second array
for (int number: positiveNumbers) {
System.out.print(number + ", ");
}
}
}
Output:
-1, 2, 3, 4, 5, 6
Here, we can see that we have changed one value of the numbers array. When we print the positiveNumbers array, we can see that the same value is also changed.
It's because both arrays refer to the same array object. This is because of the shallow copy.
Now, to make new array objects while copying the arrays, we need deep copy rather than a shallow copy.
2. Using Looping Construct to Copy Arrays
Let's take an example:
import java.util.Arrays;
class Main {
public static void main(String[] args) {
int [] source = {1, 2, 3, 4, 5, 6};
int [] destination = new int[6];
// iterate and copy elements from source to destination
for (int i = 0; i < source.length; ++i) {
destination[i] = source[i];
}
// converting array to string
System.out.println(Arrays.toString(destination));
}
}
Output:
[1, 2, 3, 4, 5, 6]
In the above example, we have used the for
loop to iterate through each element of the source array. In each iteration, we are copying elements from the source array to the destination array.
Here, the source and destination array refer to different objects (deep copy). Hence, if elements of one array are changed, corresponding elements of another array is unchanged.
Notice the statement,
System.out.println(Arrays.toString(destination));
Here, the toString() method is used to convert an array into a string.
Finding largest value in array
/**
* This program find the largest value
* in an array
*/
public class PassArray
{
public static void main(String[] args)
{
final int SIZE = 10; // Size of the array
// Create an array.
int[] list = new int[SIZE];
// Fill array with random values
for (int i = 0; i < list.length; i++)
{
list[i] = (int) (Math.random() * 100);
}
int max = 0; // hold index number of largest number
// Finding largest value.
for (int i = 0; i < list.length; i++)
{
if (list[i] > list[max])
{
max = i;
}
}
// Show all elements of array
System.out.print("Elements are : ");
for (int i = 0; i < list.length; i++)
{
System.out.print(list[i] + " ");
}
System.out.println();
// Show largest value of array
System.out.println("Largest value is " + list[max]);
}
}
Largest value is 89
7.3 Passing Array as Parameters to Methods
public static void printArray(int[] list, int n)
{
for (int i = 0; i < n; i++)
{
System.out.print(list[i] + " ");
}
}
import java.util.Scanner;
/**
* This program demonstrates passing an array as an
* argument to method.
*/
public class PassArray
{
public static void main(String[] args)
{
final int SIZE = 4; // Size of the array
// Create an array.
int[] array = new int[SIZE];
// Pass the array to the fillArray method.
fillArray(array, SIZE);
System.out.println("Numbers are :");
// Pass the array to the printArray method.
printArray(array, SIZE);
}
/**
* The fillArray method accepts an array as
* an argument. Each of its Element is filled by user.
*/
private static void fillArray(int[] list, int n)
{
// Create a Scanner object for keyboard input.
Scanner console = new Scanner(System.in);
System.out.println("Enter " + n + " integers");
for (int i = 0; i < n; i++)
{
list[i] = console.nextInt();
}
}
/**
* The printArray method accepts an array as
* an argument displays its contents.
*/
public static void printArray(int[] list, int n)
{
for (int i = 0; i < n; i++)
{
System.out.print(list[i] + " ");
}
}
}
5
8
2
9
Numbers are :
5 8 2 9
7.4 Common Array Operations
import java.util.Scanner;
/**
* This class contains common methods to
* manipulate array.
*/
public class CommonArrayMethods
{
/**
* The fillArray method accepts an array and number of
* elements as an argument. Each of its Element is filled by user.
*/
private static void fillArray(int[] list, int n)
{
// Create a Scanner object for keyboard input.
Scanner console = new Scanner(System.in);
for (int i = 0; i < n; i++)
{
list[i] = console.nextInt();
}
}
/**
* The printArray method accepts an array and number of
* elements as argument and displays its contents.
*/
public static void printArray(int[] list, int n)
{
for (int i = 0; i < n; i++)
{
System.out.print(list[i] + " ");
}
}
/**
* The sumArray method adds the sum of elements and return it.
*/
public static int sumArray(int[] list, int n)
{
int sum = 0;
for (int i = 0; i < n; i++)
{
sum += list[i];
}
return sum;
}
/**
* The largestElement method returns the index of largest element.
*/
public static int largestElement(int[] list, int n)
{
int max = 0; // store index number of first element
for (int i = 1; i < n; i++)
{
if (list[max] > list[i])
{
max = i;
}
}
return max;
}
}
public class CommonArrayMethodsDemo
{
public static void main(String[] args)
{
final int SIZE = 4; // Size of the array
// Create an array.
int[] array = new int[SIZE];
// Read into array.
System.out.println("Enter numbers ");
CommonArrayMethods.fillArray(array, SIZE);
// Print the array.
System.out.println("Numbers are :");
CommonArrayMethods.printArray(array, SIZE);
System.out.println();
// Display the sum of the elements of array.
System.out.println("The sum of the elements is: "
+ CommonArrayMethods.sumArray(array, SIZE));
// Display the index of largest element of array.
System.out.println("Largest element is at index "
+ CommonArrayMethods.largestElement(array,
SIZE));
}
}
5
8
9
2
Numbers are :
5 8 9 2
The sum of the elements is: 24
Largest element is at index 2
7.5 Returning Array from Methods
/**
* This program demonstrates how a reference to an
* array can be returned from a method.
*/
public class ReturnArray
{
public static void main(String[] args)
{
final int NUMBER = 4; // number of the elements
// Create an array variable.
int[] values;
// Values reference the array returned
// from the randomArray method.
values = randomArray(NUMBER);
// Display the values in the array.
for (int i = 0; i < values.length; i++)
{
System.out.print(values[i] + " ");
}
}
/**
* The randomArray method accept number as argument and
* returns a reference to an array of integers.
*/
public static int[] randomArray(int n)
{
int[] list = new int[n];
for (int i = 0; i < list.length; i++)
{
list[i] = (int) (Math.random() * 10);
}
return list;
}
}
7.6 Arrays of Strings
String[] fruits = new String[3];
Next, consider the statement:
fruits[0] = "Mango";
fruits[1] = "Apple";
fruits[2] = "Banana";
7.8 Two Dimensional Arrays:
datatype[][] arrayname=new datatype[rows][cols];
int[][] numbers = new int[3][5];
For example,
int[][] a = new int[3][4];
Here, we have created a multidimensional array named a. It is a 2-dimensional array, that can hold a maximum of 12 elements,
Accessing element in a two-dimensional array
numbers[1][2] = 20;
Initializing a Two Dimensional Array
int[][] values - { {10, 20, 30}, {40, 50, 60}, {70, 80, 90} };
Displaying All the Elements of a Two-Dimensional Array
/**
* This program demonstrates a two-dimensional array.
*/
public class TwoDimArray
{
public static void main(String[] args)
{
// Array of size 4x3 to hold integers.
int[][] values =
{
{ 10, 20, 30 }, { 40, 50, 60 }, { 70, 80, 90 },
{ 11, 21, 31 }
};
// Nested loops to print the array in tabular form.
for (int row = 0; row < 4; row++)
{
for (int col = 0; col < 3; col++)
{
System.out.print(values[row][col] + " ");
}
System.out.println(); // Print new line.
}
}
}
40 50 60
70 80 90
11 21 31
How to initialize a 2d array in Java?
Here is how we can initialize a 2-dimensional array in Java.
int[][] a = {
{1, 2, 3},
{4, 5, 6, 9},
{7},
};
As we can see, each element of the multidimensional array is an array itself. And also, unlike C/C++, each row of the multidimensional array in Java can be of different lengths.
Example: 2-dimensional Array
class MultidimensionalArray {
public static void main(String[] args) {
// create a 2d array
int[][] a = {
{1, 2, 3},
{4, 5, 6, 9},
{7},
};
// calculate the length of each row
System.out.println("Length of row 1: " + a[0].length);
System.out.println("Length of row 2: " + a[1].length);
System.out.println("Length of row 3: " + a[2].length);
}
}
class MultidimensionalArray {
public static void main(String[] args) {
// create a 2d array
int[][] a = {
{1, 2, 3},
{4, 5, 6, 9},
{7},
};
// calculate the length of each row
System.out.println("Length of row 1: " + a[0].length);
System.out.println("Length of row 2: " + a[1].length);
System.out.println("Length of row 3: " + a[2].length);
}
}
Output:
Length of row 1: 3 Length of row 2: 4 Length of row 3: 1
In the above example, we are creating a multidimensional array named a. Since each component of a multidimensional array is also an array (a[0]
, a[1]
and a[2]
are also arrays).
Here, we are using the length
attribute to calculate the length of each row.
Example: Print all elements of 2d array Using Loop
class MultidimensionalArray {
public static void main(String[] args) {
int[][] a = {
{1, -2, 3},
{-4, -5, 6, 9},
{7},
};
for (int i = 0; i < a.length; ++i) {
for(int j = 0; j < a[i].length; ++j) {
System.out.println(a[i][j]);
}
}
}
}
class MultidimensionalArray {
public static void main(String[] args) {
int[][] a = {
{1, -2, 3},
{-4, -5, 6, 9},
{7},
};
for (int i = 0; i < a.length; ++i) {
for(int j = 0; j < a[i].length; ++j) {
System.out.println(a[i][j]);
}
}
}
}
Output:
1 -2 3 -4 -5 6 9 7
We can also use the for...each loop to access elements of the multidimensional array. For example,
class MultidimensionalArray {
public static void main(String[] args) {
// create a 2d array
int[][] a = {
{1, -2, 3},
{-4, -5, 6, 9},
{7},
};
// first for...each loop access the individual array
// inside the 2d array
for (int[] innerArray: a) {
// second for...each loop access each element inside the row
for(int data: innerArray) {
System.out.println(data);
}
}
}
}
Output:
1 -2 3 -4 -5 6 9 7
In the above example, we are have created a 2d array named a. We then used for
loop and for...each
loop to access each element of the array.
Length field in two-dimensional array
// Nested loops to print the array in tabular form
for (int row = 0; row < values.length; row++)
{
for (int col = 0; col < values[row].length; col++)
{
System.out.print(values[row][col] + " ");
}
System.out.println(); // Print new line.
}
Common Operation in Two Dimensional Array
/**
* This class contains common methods to
* manipulate two-dimensional array.
*/
public class TwoDimArrayMethods
{
public void printMatrix(int[][] matrix)
{
for (int row = 0; row < matrix.length; row++)
{
for (int col = 0; col < matrix[row].length; col++)
{
System.out.printf("%7d", matrix[row][col]);
}
System.out.println();
}
}
public void sumRows(int[][] matrix)
{
int sum;
// sum of each individual row
for (int row = 0; row < matrix.length; row++)
{
sum = 0;
for (int col = 0; col < matrix[row].length; col++)
{
sum = sum + matrix[row][col];
}
System.out.println("The sum of row " + (row + 1) + " = "
+ sum);
}
}
public void largestInRows(int[][] matrix)
{
int largest;
for (int row = 0; row < matrix.length; row++)
{
// assume that the first element of the row is largest
largest = matrix[row][0];
for (int col = 1; col < matrix[row].length; col++)
{
if (largest < matrix[row][col])
{
largest = matrix[row][col];
}
}
System.out.println("The largest element of row " + (row + 1)
+ " = " + largest);
}
}
}
public class TwoDimArrayMethodsDemo
{
public static void main(String[] args)
{
TwoDimArrayMethods operate = new TwoDimArrayMethods();
int[][] board =
{
{ 20, 15, 6, 19, 18 }, { 4, 46, 24, 17, 18 },
{ 12, 50, 23, 16, 31 }
};
operate.printMatrix(board);
System.out.println();
operate.sumRows(board);
System.out.println();
operate.largestInRows(board);
}
}
20 15 6 19 18 4 46 24 17 18 12 50 23 16 31 The sum of row 1 = 78 The sum of row 2 = 109 The sum of row 3 = 132 The largest element of row 1 = 20 The largest element of row 2 = 46 The largest element of row 3 = 50
inserting the data into two dimensional array:
arrayname[rowindex][colindex]=value;
//firstrow
str[0][0]="rc";
str[0][1]="ide";
//2ndrow
str[1][0]="wd";
str[1][1]="selenium";
//3rd row
str[2][0]="java";
str[2][1]="ruby";
how to find the number of rows in array
int r=arrayname.length;
how to find the number of columns in array
int c=arrayname[0].length;
//printing two dimensional array
for(int i=0;i<r;i++){ //0<3-T/i=0+1=1/1<3=T/i=1+1=2/2<3-T/i=2+1=3/3<3-F
for(int j=0;j<c;j++){/0<2-T/j=0+1=1/1<2-T/j=1+1=2/2<2=F/j=0,0<2-T/j=0+1=1/1<2=T/j=1+1=2/2<2-F///0<2-T/j=0+1-1/1<2-T/j=1+1=2/2<2=F
System.out.print(str[i][j]+"\t");/str[0][0]/str[0][1]/str[1][0], str[1][1]//str[2][0] str[2][1]
}
System.out.println();
}
No comments:
Post a Comment
Note: Only a member of this blog may post a comment.