Both RestAssured and Karate are popular frameworks for API testing, but they serve slightly different purposes and have different strengths and weaknesses. Here’s a comparison to help you decide which one might be better for your specific needs:
RestAssured
Pros:
- Java Integration: RestAssured is a Java-based library, making it a good choice if you're already working within a Java ecosystem. It can easily integrate with other Java tools and frameworks.
- Flexibility: RestAssured provides fine-grained control over HTTP requests and responses, which is useful for complex testing scenarios.
- Mature and Robust: It is a well-established tool with extensive documentation and a large user community.
- Support for BDD: RestAssured supports Behavior-Driven Development (BDD) style tests using Cucumber.
Cons:
- Verbose: Writing tests can be more verbose compared to Karate, as you need to write more code to achieve the same functionality.
- Steeper Learning Curve: For beginners, getting started with RestAssured might be more challenging due to its extensive configuration and setup requirements.
Karate
Pros:
- Ease of Use: Karate is designed to be simple and easy to use, with a low learning curve. It uses a domain-specific language (DSL) that is very readable and concise.
- All-in-One Framework: Karate integrates API testing, mocking, performance testing, and UI testing (via Selenium) into a single framework.
- No Coding Required: You can write tests without having to write any Java code, making it accessible for non-developers.
- Built-in Assertions: Karate comes with built-in support for JSON and XML assertions, which makes it very convenient for validating responses.
Cons:
- Less Flexibility: While Karate is very powerful for most standard API testing needs, it might lack the flexibility required for more complex testing scenarios compared to RestAssured.
- Limited Customization: Being a higher-level framework, it might not provide as much control over the HTTP requests and responses as RestAssured.
- Performance: For extremely large and complex test suites, RestAssured might perform better due to its fine-grained control and optimizations.
When to Use RestAssured
- Java Ecosystem: If your project is heavily based on Java and you prefer writing tests in Java.
- Complex Scenarios: When you need extensive control over your HTTP requests and responses.
- BDD Support: If you want to integrate with Cucumber for BDD-style tests.
When to Use Karate
- Simplicity and Speed: When you need to get started quickly with minimal setup and want to write readable, maintainable tests.
- Non-Developers: If your testing team includes members who are not proficient in coding.
- All-in-One Solution: When you want a single framework to handle API, UI, and performance testing without needing multiple tools.
Conclusion
Both frameworks have their strengths and can be the better choice depending on your specific requirements. If you are looking for ease of use, quick setup, and an all-in-one testing solution, Karate might be the better choice. On the other hand, if you need more control, flexibility, and integration with a Java ecosystem, RestAssured would be more suitable.
Evaluate your project needs, team skillset, and the complexity of your test scenarios to make the best choice.
=========================================
Why API Automation is better than UI Automation?
API automation is the process of automating interactions with Application Programming Interfaces (APIs) using scripts, tools, or software.
UI automation, or User Interface automation, refers to the process of using software or tools to simulate user interactions with a graphical user interface (GUI) of a software application or system.

Here are some reasons why API automation is considered better than UI automation in certain scenarios:
- Faster Execution:
API tests tend to run faster than UI tests because they don’t involve rendering web pages or interacting with the user interface. API requests and responses are typically lightweight, leading to quicker test execution.
- Stability:
UI tests can be fragile and sensitive to changes in the user interface, making them prone to breakage when UI elements or layouts change. API tests are generally more stable because they focus on the underlying business logic and data flow.
- Coverage:
API tests can provide comprehensive coverage of the application’s functionality, including edge cases and error scenarios. UI tests may not cover all possible user interactions or inputs.
- Efficiency:
API tests can be more efficient for repetitive tasks like regression testing or load testing because they require fewer resources and can be run in parallel.
- Data Validation:
API tests are well-suited for data validation because they can easily verify the correctness of data returned by the API. UI tests may require additional effort to extract and validate data displayed on the user interface.
- Cross-Platform and Cross-Browser Compatibility:
API tests are not dependent on specific browsers or platforms, making them more suitable for testing the backend of applications that need to work across different environments.
- Early Detection of Issues:
API tests can be integrated into the development pipeline to catch issues early in the development process, promoting a shift-left approach to testing.
- Reduced Maintenance:
UI automation scripts often require frequent updates due to changes in the user interface. API tests tend to have lower maintenance overhead since they are less affected by UI changes.
- Scalability:
API automation can easily scale to test multiple endpoints, versions, or services in a micro-services architecture.
- Isolation:
API tests can be isolated from the user interface, allowing you to test specific functionalities or endpoints independently. This isolation simplifies debugging and makes it easier to identify the root cause of issues.
Let’s illustrate the difference between API automation and UI automation using a simple scenario of testing a login functionality.

I am using two popular frameworks; Postman for API automation & Selenium for UI automation.
API Automation Example (Using Postman)
In this API automation example, we’re using Postman to send a POST request to a login API endpoint. We then assert that the HTTP status code is 200 and that the response contains a “success” attribute set to true
.
// Postman test script for API automation
pm.test("Login API Test", function () {
// Define the API endpoint and request parameters
const url = "https://api.example.com/login";
const request = {
method: "POST",
url: url,
header: {
"Content-Type": "application/json",
},
body: {
mode: "raw",
raw: JSON.stringify({
username: "testuser",
password: "password123",
}),
},
};
// Send the API request
const response = pm.sendRequest(request);
// Perform assertions on the API response
pm.expect(response.code).to.equal(200); // Check HTTP status code
pm.expect(response.json().success).to.be.true; // Check a specific JSON attribute
});
UI Automation Example (Using Selenium and Python)
In this UI automation example, we’re using Selenium (with Python) to automate interactions with a web-based login page. We locate elements on the page, enter login credentials, click the login button, then verify the presence of a welcome message to confirm successful login.
from selenium import webdriver
# Set up the Selenium WebDriver for a web browser (e.g., Chrome)
driver = webdriver.Chrome()
# Open the login page
driver.get("https://www.example.com/login")
# Locate the username and password input fields and the login button
username_input = driver.find_element_by_id("username")
password_input = driver.find_element_by_id("password")
login_button = driver.find_element_by_id("login-button")
# Enter login credentials and submit the form
username_input.send_keys("testuser")
password_input.send_keys("password123")
login_button.click()
# Perform assertions on the UI to verify successful login
welcome_message = driver.find_element_by_css_selector(".welcome-message")
assert "Welcome, testuser!" in welcome_message.text
# Close the browser
driver.quit()
As you can see, API automation focuses on making HTTP requests and validating API responses, while UI automation interacts with the user interface of a web application.
Summary
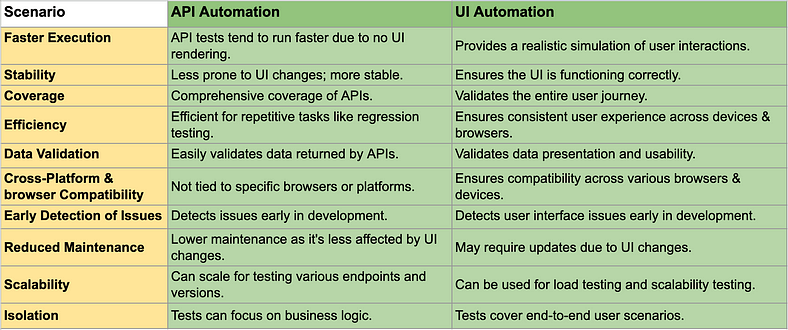
No comments:
Post a Comment
Note: Only a member of this blog may post a comment.