PRG-1)Welcome to the world of Java! In this challenge, we practice printing to stdout.
The code stubs in your editor declare a Solution class and a main method. Complete the main method by copying the two lines of code below and pasting them inside the body of your main method.
System.out.println("Hello, World.");
System.out.println("Hello, Java.");
Input Format
There is no input for this challenge.
Output Format
You must print two lines of output:
- Print
Hello, World.
on the first line. - Print
Hello, Java.
on the second line.
Sample Output
Hello, World.
Hello, Java.
================================================================
PRG-2)
In this challenge, we test your knowledge of using if-else conditional statements to automate decision-making processes. An if-else statement has the following logical flow:
Source: Wikipedia
Task
Given an integer,, perform the following conditional actions:
- If is odd, print
Weird
- If is even and in the inclusive range of to , print
Not Weird
- If is even and in the inclusive range of to , print
Weird
- If is even and greater than , print
Not Weird
Complete the stub code provided in your editor to print whether or not is weird.
Input Format
A single line containing a positive integer, .
Constraints
Output Format
Print Weird
if the number is weird; otherwise, print Not Weird
Sample Input 0
3
Sample Output 0
Weird
Sample Input 1
24
Sample Output 1
Not Weird
Explanation
Sample Case 0:
is odd and odd numbers are weird, so we print Weird
.
Sample Case 1:
and is even, so it isn't weird. Thus, we print Not Weird
.
-----------------------------------------------------------------------------------------------------------------
PRG-3)
In this challenge, you must read an integer, a double, and a String from stdin, then print the values according to the instructions in the Output Format section below. To make the problem a little easier, a portion of the code is provided for you in the editor.
Note: We recommend completing Java Stdin and Stdout I before attempting this challenge.
Input Format
There are three lines of input:
- The first line contains an integer.
- The second line contains a double.
- The third line contains a String.
Output Format
There are three lines of output:
- On the first line, print
String:
followed by the unaltered String read from stdin. - On the second line, print
Double:
followed by the unaltered double read from stdin. - On the third line, print
Int:
followed by the unaltered integer read from stdin.
To make the problem easier, a portion of the code is already provided in the editor.
Note: If you use the nextLine() method immediately following the nextInt() method, recall that nextInt() reads integer tokens; because of this, the last newline character for that line of integer input is still queued in the input buffer and the next nextLine() will be reading the remainder of the integer line (which is empty).
Sample Input
42
3.1415
Welcome to HackerRank's Java tutorials!
Sample Output
String: Welcome to HackerRank's Java tutorials!
Double: 3.1415
Int: 42
Sample Code:
import java.util.Scanner;
public class Solution {
public static void main(String[] args) { Scanner scan = new Scanner(System.in); int i = scan.nextInt(); // Write your code here. System.out.println("String: " + s); System.out.println("Double: " + d); System.out.println("Int: " + i); }}
=======================================================================
PRG-4.
Consider the following problem:
Write a short program that prints each number from 1 to 100 on a new line.
For each multiple of 3, print "Fizz" instead of the number.
For each multiple of 5, print "Buzz" instead of the number.
For numbers which are multiples of both 3 and 5, print "FizzBuzz" instead of the number.
====================================================================
PRG-5)write a program to check given number is
Perfect Number or not in Java?
A perfect number is a number that is equal to the sum of its positive divisors (excluding the number itself). For example, 6, 28, 496 etc. are perfect numbers. Because:
6 = 1+2+3 = 6where 1, 2, and 3 are positive divisors of 6. Similarly:
28 = 1+2+4+7+14 = 28
PRG-6)
write a Java program to convert days into seconds. The days must be received by user at run-time
PRG-7)Write a Java Program to Count Total Number of Digits in a Number?
PRG-8)Write a Java Program to Check Leap Year or Not.
A leap year is an year that
- is divisible by 4, but not by 100
- or is divisible by 400
- Find largest of two numbers using if...else
- Find largest of two numbers using conditional operator[ternary operator]
- Find largest of two numbers using user-defined function
PRG-17)In this challenge, we're going to use loops to help us do some simple math.
Task
Given an integer, , print its first multiples. Each multiple (where ) should be printed on a new line in the form: N x i = result
.
Input Format
A single integer, .
Constraints
Output Format
Print lines of output; each line (where ) contains the of in the form:N x i = result
.
Sample Input
2
Sample Output
2 x 1 = 2
2 x 2 = 4
2 x 3 = 6
2 x 4 = 8
2 x 5 = 10
2 x 6 = 12
2 x 7 = 14
2 x 8 = 16
2 x 9 = 18
2 x 10 = 20
=====================================================================
PRG-18)
Java's System.out.printf function can be used to print formatted output. The purpose of this exercise is to test your understanding of formatting output using printf.
To get you started, a portion of the solution is provided for you in the editor; you must format and print the input to complete the solution.
Input Format
Every line of input will contain a String followed by an integer.
Each String will have a maximum of alphabetic characters, and each integer will be in the inclusive range from to .
Output Format
In each line of output there should be two columns:
The first column contains the String and is left justified using exactly characters.
The second column contains the integer, expressed in exactly digits; if the original input has less than three digits, you must pad your output's leading digits with zeroes.
Sample Input
java 100
cpp 65
python 50
Sample Output
================================
java 100
cpp 065
python 050
================================
Explanation
Each String is left-justified with trailing whitespace through the first characters. The leading digit of the integer is the character, and each integer that was less than digits now has leading zeroes.
======================================================================="PRG-19)
In computing, End Of File (commonly abbreviated EOF) is a condition in a computer operating system where no more data can be read from a data source." — (Wikipedia: End-of-file)
The challenge here is to read lines of input until you reach EOF, then number and print all lines of content.
Hint: Java's Scanner.hasNext() method is helpful for this problem.
Input Format
Read some unknown lines of input from stdin(System.in) until you reach EOF; each line of input contains a non-empty String.
Output Format
For each line, print the line number, followed by a single space, and then the line content received as input.
Sample Input
Hello world
I am a file
Read me until end-of-file.
Sample Output
1 Hello world
2 I am a file
3 Read me until end-of-file.
=========================================================================================================================================================
PRG-20)
Static initialization blocks are executed when the class is loaded, and you can initialize static variables in those blocks.
It's time to test your knowledge of Static initialization blocks. You can read about it here.
You are given a class Solution with a main method. Complete the given code so that it outputs the area of a parallelogram with breadth and height . You should read the variables from the standard input.
If or , the output should be "java.lang.Exception: Breadth and height must be positive" without quotes.
Input Format
There are two lines of input. The first line contains : the breadth of the parallelogram. The next line contains : the height of the parallelogram.
Constraints
Output Format
If both values are greater than zero, then the main method must output the area of the parallelogram. Otherwise, print "java.lang.Exception: Breadth and height must be positive" without quotes.
Sample input 1
1
3
Sample output 1
3
Sample input 2
-1
2
Sample output 2
java.lang.Exception: Breadth and height must be positive
============================================================================================================
PRG-21)
You are given an integer , you have to convert it into a string.
Please complete the partially completed code in the editor. If your code successfully converts into a string the code will print "Good job". Otherwise it will print "Wrong answer".
can range between to inclusive.
Sample Input 0
100
Sample Output 0
Good job
=========================================================
PRG-22)
The Calendar class is an abstract class that provides methods for converting between a specific instant in time and a set of calendar fields such as YEAR, MONTH, DAY_OF_MONTH, HOUR, and so on, and for manipulating the calendar fields, such as getting the date of the next week.
You are given a date. You just need to write the method, getDay, which returns the day on that date. To simplify your task, we have provided a portion of the code in the editor.
Example:
month = 8day = 14year = 2017The method should return MONDAY as the day on that date.
Function Description
Complete the findDay function in the editor below.
findDay has the following parameters:
- int: month
- int: day
- int: year
Returns
- string: the day of the week in capital letters
Input Format
A single line of input containing the space-separated month, day and year, respectively, in MM DD YYYY format.
Constraints
- 2000 < year < 3000
Sample Input:
08 05 2015
Sample Output:
WEDNESDAY
==============================================================
PRG-23)
Given a double-precision number, payment, denoting an amount of money, use the NumberFormat class' getCurrencyInstance method to convert the payment into the US, Indian, Chinese, and French currency formats. Then print the formatted values as follows:
US: formattedPayment
India: formattedPayment
China: formattedPayment
France: formattedPayment
where formattedPayment is payment formatted according to the appropriate Locale's currency.
Note: India does not have a built-in Locale, so you must construct one where the language is en (i.e., English).
Input Format
A single double-precision number denotes payment.
Constraints
- 0 <= payment <= 10^9
Output Format
On the first line, print US: u where u is payment formatted for US currency.On the second line, print India: i where i is payment formatted for the Indian currency.On the third line, print China: c where c is payment formatted for Chinese currency.On the fourth line, print France: f, where f is payment formatted for French currency.
Sample Input
12324.134
Sample Output
US: $12,324.13
India: Rs.12,324.13
China: ¥12,324.13
France: 12 324,13 €
Explanation
Each line contains the value of payment formatted according to the four countries' respective currencies.=============================================================================================================PRG-24"A string is traditionally a sequence of characters, either as a literal constant or as some kind of variable." — Wikipedia: String (computer science)
This exercise is to test your understanding of Java Strings. A sample String declaration:
String myString = "Hello World!"
The elements of a String are called characters. The number of characters in a string is called the length, and it can be retrieved with the String.length() method.
Given two strings of lowercase English letters, A and B, perform the following operations:- Sum the lengths of A and B.
- Determine if A is lexicographically larger than B (i.e.: does B come before A in the dictionary?).
- Capitalize the first letter in A and B and print them on a single line, separated by a space.
Input Format
The first line contains a string A. The second line contains another string B. The strings are comprised of only lowercase English letters.
Output Format
There are three lines of output:For the first line, sum the lengths A of and B.For the second line, write Yes if A is lexicographically greater than B otherwise print No instead.For the third line, capitalize the first letter in both A and B and print them on a single line, separated by a space.
Sample Input
hello
java
Sample Output
9
No
Hello Java
Explanation
String A is "hello" and B is "java".
A has a length of 5, and B has a length of 4; the sum of their lengths is 9.When sorted alphabetically/lexicographically, "hello" precedes "java"; therefore, A is not greater than B and the answer is No.
When you capitalize the first letter of both A and B and then print them separated by a space, you get "Hello Java".
=========================================================================PRG-25Write a Java program to find the length of the last word in a given string?g
Input:String s="welcome to java"
Output4 ==============================================================PRG-26Java has 8 primitive data types; char, boolean, byte, short, int, long, float, and double. For this exercise, we'll work with the primitives used to hold integer values (byte, short, int, and long):
- A byte is an 8-bit signed integer.
- A short is a 16-bit signed integer.
- An int is a 32-bit signed integer.
- A long is a 64-bit signed integer.
Given an input integer, you must determine which primitive data types are capable of properly storing that input.
To get you started, a portion of the solution is provided for you in the editor.
Reference: https://docs.oracle.com/javase/tutorial/java/nutsandbolts/datatypes.html
Input Format
The first line contains an integer, , denoting the number of test cases.
Each test case, , is comprised of a single line with an integer, , which can be arbitrarily large or small.
Output Format
For each input variable and appropriate primitive , you must determine if the given primitives are capable of storing it. If yes, then print:
n can be fitted in:
* dataType
If there is more than one appropriate data type, print each one on its own line and order them by size (i.e.: ).
If the number cannot be stored in one of the four aforementioned primitives, print the line:
n can't be fitted anywhere.
Sample Input
5
-150
150000
1500000000
213333333333333333333333333333333333
-100000000000000
Sample Output
-150 can be fitted in:
* short
* int
* long
150000 can be fitted in:
* int
* long
1500000000 can be fitted in:
* int
* long
213333333333333333333333333333333333 can't be fitted anywhere.
-100000000000000 can be fitted in:
* long
Explanation
can be stored in a short, an int, or a long.
is very large and is outside of the allowable range of values for the primitive data types discussed in this problem.
------------------------------------------------------------------------------------
import java.util.*;import java.io.*;class Solution{ public static void main(String []argh) { Scanner sc = new Scanner(System.in); int t=sc.nextInt();
for(int i=0;i<t;i++) {
try { long x=sc.nextLong(); System.out.println(x+" can be fitted in:"); if(x>=-128 && x<=127)System.out.println("* byte"); //write remaining code here } catch(Exception e) { System.out.println(sc.next()+" can't be fitted anywhere."); }
} }}
================================================
PRG-27Two strings, and , are called anagrams if they contain all the same characters in the same frequencies. For this challenge, the test is not case-sensitive. For example, the anagrams of CAT
are CAT
, ACT
, tac
, TCA
, aTC
, and CtA
.
Function Description
Complete the isAnagram function in the editor.
isAnagram has the following parameters:
- string a: the first string
- string b: the second string
Returns
- boolean: If and are case-insensitive anagrams, return true. Otherwise, return false.
Input Format
The first line contains a string .
The second line contains a string .
Constraints
- Strings and consist of English alphabetic characters.
- The comparison should NOT be case sensitive.
Sample Input 0
anagram
margana
Sample Output 0
Anagrams
Explanation 0
Character Frequency: anagram
Frequency: margana
A
or a
3 3 G
or g
1 1 N
or n
1 1 M
or m
1 1 R
or r
1 1
The two strings contain all the same letters in the same frequencies, so we print "Anagrams".
Sample Input 1
anagramm
marganaa
Sample Output 1
Not Anagrams
Explanation 1
Character Frequency: anagramm
Frequency: marganaa
A
or a
3 4 G
or g
1 1 N
or n
1 1 M
or m
2 1 R
or r
1 1
The two strings don't contain the same number of a
's and m
's, so we print "Not Anagrams".
Sample Input 2
Hello
hello
Sample Output 2
Anagrams
Explanation 2
Character Frequency: Hello
Frequency: hello
E
or e
1 1 H
or h
1 1 L
or l
2 2 O
or o
1 1
The two strings contain all the same letters in the same frequencies, so we print "Anagrams".
============================================================
PRG-28-Inheritance
Using inheritance, one class can acquire the properties of others. Consider the following Animal class:
class Animal{
void walk(){
System.out.println("I am walking");
}
}
This class has only one method, walk. Next, we want to create a Bird class that also has a fly method. We do this using extends keyword:
class Bird extends Animal {
void fly() {
System.out.println("I am flying");
}
}
Finally, we can create a Bird object that can both fly and walk.
public class Solution{
public static void main(String[] args){
Bird bird = new Bird();
bird.walk();
bird.fly();
}
}
The above code will print:
I am walking
I am flying
This means that a Bird object has all the properties that an Animal object has, as well as some additional unique properties.
The code above is provided for you in your editor. You must add a sing method to the Bird class, then modify the main method accordingly so that the code prints the following lines:
I am walking
I am flying
I am singing
===================================================
PRG-29 -INHERITANCE-II
Write the following code in your editor below:
- A class named Arithmetic with a method named add that takes integers as parameters and returns an integer denoting their sum.
- A class named Adder that inherits from a superclass named Arithmetic.
Your classes should not be be .
Input Format
You are not responsible for reading any input from stdin; a locked code stub will test your submission by calling the add method on an Adder object and passing it integer parameters
Output Format
You are not responsible for printing anything to stdout. Your add method must return the sum of its parameters.
Sample Output
The main method in the Solution class above should print the following:
My superclass is: Arithmetic
42 13 20
=======================================================
PRG-30-
Write a Program to remove the given character from a
String
Input:
String s="Automation";
char c='o'
Output:
==============================
Autmatin
PRG-31--
Exercise 31 - Life in Weeks
------------------------------------------
Instructions
I was reading this article by Tim Urban - Your Life in Weeks and realised just how little time we actually have.
https://waitbutwhy.com/2014/05/life-weeks.html
Create a program using maths and f-Strings that tells us how many days, weeks, months we have left if we live until 90 years old.
It will take your current age as the input and output a message with our time left in this format:
You have x days, y weeks, and z months left.
Where x, y and z are replaced with the actual calculated numbers.
Warning your output should match the Example Output format exactly, even the positions of the commas and full stops.
Hint
- There are 365 days in a year, 52 weeks in a year and 12 months in a yea
Example Input
56
Example Output
You have 12410 days, 1768 weeks, and 408 months left.
PRG-32
Exercise 32 - BMI Calculator
Instructions
Write a program that calculates the Body Mass Index (BMI) from a user's weight and height.
The BMI is a measure of someone's weight taking into account their height. e.g. If a tall person and a short person both weigh the same amount, the short person is usually more overweight.
The BMI is calculated by dividing a person's weight (in kg) by the square of their height (in m):
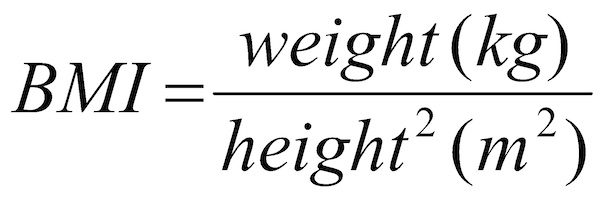
Warning you should convert the result to a whole number.
Hint
- Check the data type of the inputs.
- Try to use the exponent operator in your code.
- Remember PEMDAS.
- Remember to convert your result to a whole number (int).
Example Input
weight = 80
height = 1.75
Example Output
80 ÷ (1.75 x 1.75) = 26.122448979591837
26
===========================================================================PRG-33:Task
The provided code stub reads two integers from STDIN, and . Add code to print three lines where:
- The first line contains the sum of the two numbers.
- The second line contains the difference of the two numbers (first - second).
- The third line contains the product of the two numbers.
Example
Print the following:
8
-2
15
======================================================
PRG-34 : Task
The provided code stub reads and integer, , from STDIN. For all non-negative integers , print .
Example
The list of non-negative integers that are less than is . Print the square of each number on a separate line.
0
1
4
Input Format
The first and only line contains the integer, .
Constraints
Output Format
Print lines, one corresponding to each .
Sample Input 0
5
Sample Output 0
0
1
4
9
16
No comments:
Post a Comment
Note: Only a member of this blog may post a comment.