Playwright is a popular JavaScript library for
automating web applications. With Playwright, we can write automated
tests for web applications using JavaScript, TypeScript, or any other
language.
One of the best practices for writing maintainable and scalable tests
is to use the Page Object Model (POM) pattern. In this blog, we’ll
learn how to create a Playwright JavaScript POM framework from scratch.
The Playwright POM framework offers several benefits, including:
- Modularity: The framework enables the creation of modular and reusable tests that can be easily maintained and extended.
- Readability: The use of Page Objects makes test
scripts more readable and easier to understand as the logic for
interacting with web elements is abstracted away.
- Scalability: The framework can be used to create tests for any number of web pages or applications, making it highly scalable.
- Maintainability: The framework enables test scripts to be easily updated and maintained, reducing the risk of regression issues.
Website and its module for testing
In our Playwright JavaScript framework, we automated several tasks on products page and product detail page the https://automationexercise.com/ site. These included:
- Placing an Order: We automated the process of
selecting a product, adding it to the cart, filling out the necessary
shipping and payment details, and placing the order to ensure that the
e-commerce functionality of the website is working as intended.
- Product Detail Verification and Cross test with API response:
We automated the process of verifying product details by navigating to a
product page, ensuring that the correct information is displayed,
Validating API response and UI response ( product list is showing the
same), and validating the total product count.
To ensure that our API calls were functioning correctly, we integrated API testing
with our UI testing. This allowed us to verify that the data displayed
on the website is accurate and that the website is communicating with
the API correctly.
Additionally, we included a test case for visual testing. Visual testing involves comparing screenshots
of the website before and after a change is made to ensure that the
change did not cause any unintended visual issues. By including visual
testing in our framework, we were able to catch any visual bugs early in
the development process and ensure that the website looks and functions
correctly for users
To simplify our automation efforts, we used already saved login details that allowed us to quickly log in to the site and perform the necessary tasks without having to manually enter login credentials each time.
By automating these tasks, we were able to save time and ensure that
the website functions correctly across different scenarios.
Pre-requisites
Before we start, you’ll need to have some prerequisites:
- Node.js and npm installed on your system.
- Basic knowledge of JavaScript and playwright test automation.
Setup
First, let’s create a new directory for our project and initialize it
with npm. Open your terminal or command prompt and run the following
commands:
mkdir playwright-pom-framework
cd playwright-pom-framework npm init -y
This will create a new directory called playwright-pom-framework,
change the current directory to it, and initialize it with npm.
The -y flag is used to accept the default values for the package.json file.
Next, let’s install Playwright as a development dependency:
npm install playwright –save-dev
Folder Structure
Now that we’ve set up the project, let’s create the folder structure for our framework.

playwright-pom-framework/
├── Data/
└── login_data.js and product data page
├── pages/
│ └── LoginPage.js and other pages
├── tests/
│ ├── ValidatePlaceOrder.spec.js
│ └── ValidateProductDetails.spec.js
├── config/
│ └── playwright.config.js
├── reports/
│ ├── html/ └──Allure Reports
├── global-setup.js/
├── testconfig.js/
└── package.json
Here’s a brief description of each folder:
- pages/: This folder will contain the page
objects for our application. Each page object will have methods and
properties that represent the page’s elements and actions.
- tests/: This folder will contain the test files for our application. Each test file will use the page objects to perform the tests.
- playwright.config.js: This folder will
contain the configuration file for our framework. We’ll use this file to
specify the settings for Playwright and our reporting tools.
- reports/: This folder will contain the reports generated by our tests.
- Data/: This folder contains the test data require for testing.
- global-setup.js/: Setup file to set before initialising the test.
- Testconfig.js: This file contains the different environment uri ( Web and API )
Configuration File
Now, let’s create the playwright.config.js file. This file will
contain the configuration settings for Playwright, Browser
configuration, and our reporting tools. Here’s an example configuration.
Configuration Options
Here are some of the configuration options available in the testConfig file:
- timeout: Sets the timeout for each test
case, in milliseconds. If a test case takes longer than this time to
complete, it will be marked as failed.
- retries: Sets the number of times to retry a failed test case before marking it as failed.
- reporter: Sets the reporter to use for test results. Playwright supports several built-in reporters, including dot, list, and junit.
- projects: Allows running tests in multiple browser configurations or environments.
he projects option is a powerful feature of Playwright that allows
you to run the same tests in different browser configurations or
environments. For example, you could run your tests on both desktop and
mobile devices, or on different versions of the same browser.

Pages
In the POM design pattern, each web page of the application is
represented by a Page Object Model. A Page Object Model is a JavaScript
class that defines the elements and actions on the page. Here’s an
example of a LoginPage Page Object Model.
Pages We implement:
LoginPage.js
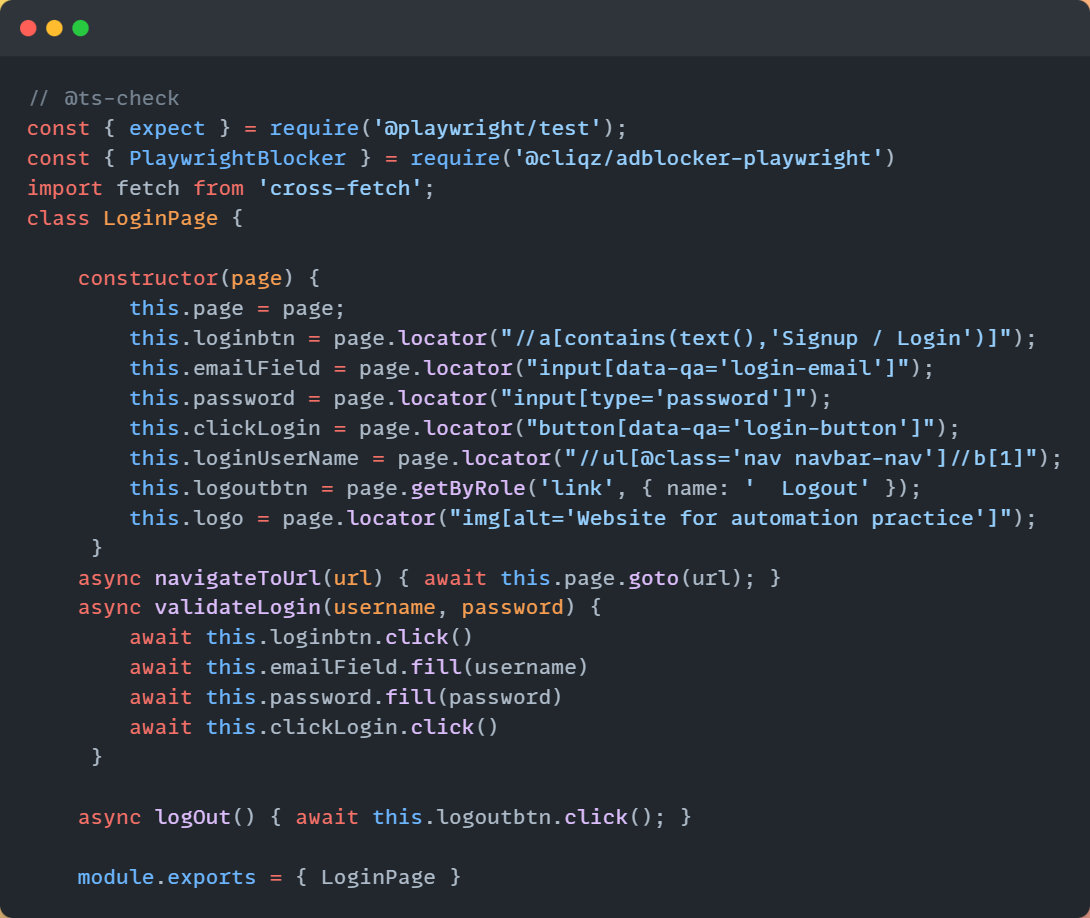
In Loginpage:
- Navigate to URL method
- Login to Website
- Validate the Company Logo ( Visual Test )
DashboardPage.js

In DashboardPage:
- Added method to search product by its name and add to cart
- Click on Cart Button
CartPage.js

In CartPage.js
- Verify the correct product is added
- Click on Checkout button
PaymentPage.js

In PaymentPage.js
- Added address input method
- Clicking on placeorder button
CheckOutpage.js

In CheckOutPage.js
- We verify the address detail
- Click on placeOrder
ConfirmationPage.js

In ConfirmationPage.js
- We validate the confirmation text
ProductsPage.js

In productsPage we validate:
- Navigate to product page ( as we are in login state )
- Validate total product available in the product page.
- Validate product UI data and API data
- Validate Search
- Validate product detail
Final page we have pomanager

The POManager class contains references to all the other page classes
defined in the code.Each page class (LoginPage, DashboardPage,
CartPage, etc.) represents a different page of the web application, and
contains methods and UI locators specific to
that page. The POManager class initializes instances of all these page
classes, passing the main page object and request object to each of
them.
Finally, the POManager class defines getter methods for each of the page classes, which can be used in the test scripts to access the methods and locators specific to each page.
This way, the test scripts can interact with the UI elements and
perform actions on the web pages using the methods defined in the
individual page classes, without worrying about the low-level details of
locating those elements.
global-setup File
The goblal-setup.js file is used in our Playwright JavaScript framework to automate the login process for the website.
This file is run before all tests to log in and store the login state in a state.json and also delete a previously generated allure reports file. By doing so, we can ensure that the website remains in a logged-in state throughout the testing process, which saves time and improves testing efficiency.
Overall, the goblal-setup.js file is a key
component of our automation framework, and its use ensures that we can
test the website thoroughly and efficiently.

Global-teardown.js
The global-teardown.js is a class in
Playwright that runs after all test cases have finished running. In this
class, we can perform any cleanup or teardown tasks that we need to do
after the tests are finished.
In the specific example you provided, global-teardown.js is used to store the Playwright report in a zip folder so that it can be easily shared with others. This is done using the Adm-zip npm module, which allows us to create and manipulate zip archives.

Test Files/Test cases:
- Validate the add product to cart and validate the product details
- Validate placeorder

In the above code we are placing order with multiple product and validating the correct product is ordered.
Testcase:
TC_04 Validate the Product count
TC_05 Validate API response with UI
TC_06 Verify that on the product detail page detail is visible:
product ProductName, category, price, availability, condition, brand
TC_07 Verify all the products related to search are visible

In the products page, we validate the total products count. Later, we
extracted the api product detail and match with the UI and also match
the product details.
Running Script:
In package.json file we create some script so we can run our test
cases according to our need like smoke test, running single test,
running parallel test

“scripts”: {
“test”: “npx playwright test”,
“single”: “npx playwright test login_state.spec.js”,
“test:single”: “npx cross-env ENV=qa npx playwright test TC_08_Verify_All_Products_and_product_detail_page.spec.js –project=Chrome”,
“test:parallel”: “npx playwright test –grep @Smoke –project=Chrome”,
“test:serial”: “npx playwright test –grep @Smoke –workers=1 –project=Chrome”,
“test:smoke”: “npx playwright test –grep @Smoke –workers=1 –project=Chrome”,
“test:api”: “npx playwright test –grep @API –workers=1 –project=Chrome”,
“test:record”: “npx playwright codegen https://automationexercise.com”,
“test:visual”: “npx playwright test VerifyLogo.spec.js –project=Chrome”,
“test:device”: “npx playwright test TC_08_Verify_All_Products_and_product_detail_page.spec.js –project=Device”,
“api”: “npx playwright test test_api.spec.js”,
“report”: “npx playwright show-report”,
“debug”: “npx playwright test TC_01_Login_User.spec.js –debug”,
“multidata”: “npx playwright test multi_data_test.spec.js”, “test:e2e”: “npx cross-env ENV=qa npx playwright test –grep @e2e –workers=1 –project=Chrome”
Example:
- Running all test scripts : – npx cross-env ENV={environment under test} npm run test
- Running single test script:- npx cross-env ENV={environment under test} npm run test:single
- Running smoke tag test script:– npx cross-env ENV={environment under test} npm run test:smoke
Reporting:
We use playwright default html report and allure reports


Allure Report:
When implementing Playwright POM framework, integrating Allure
reporting provides detailed reports with customizable features and
advanced analytics. It offers easy-to-read dashboards and seamless
integration with other popular frameworks like JUnit and TestNG.
Overall, integrating Allure reporting into your Playwright POM framework
helps optimize testing efforts and improve software quality.
The Reports are store in the my-allure-reports folder
And we can access this using command “allure serve my-allure-reports”

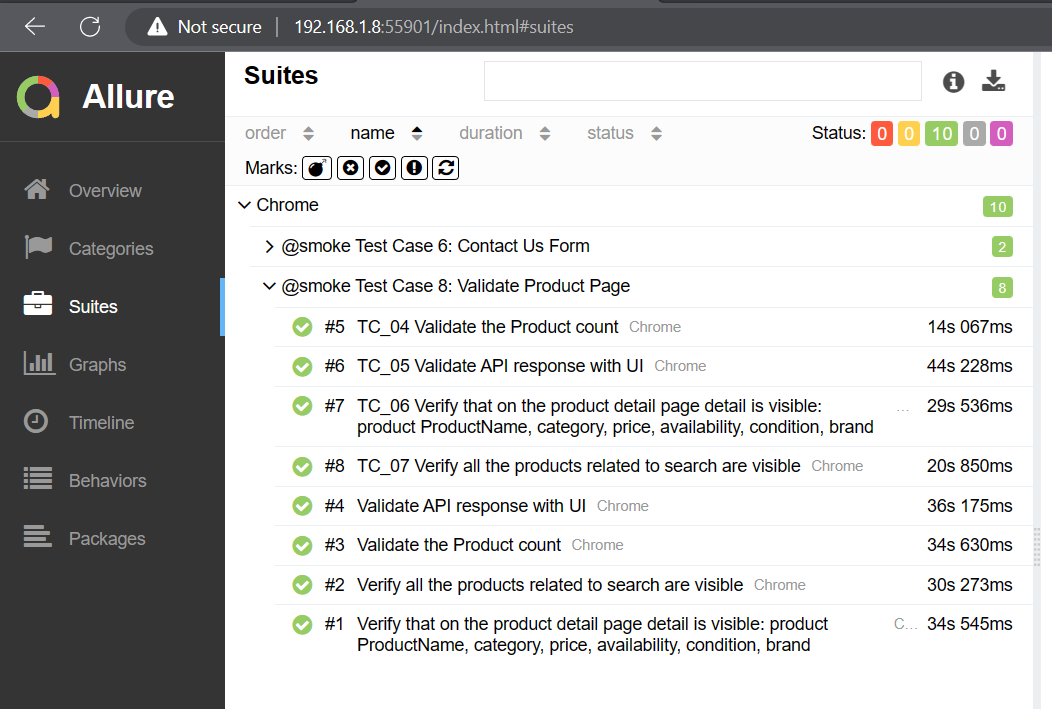
Conclusion
In this article, we discussed how to build a Playwright JavaScript
framework using the Page Object Model (POM). We defined a folder
structure, set up configuration data, created a Playwright class, and
discussed reporting tools. By following these guidelines, you can build a
robust and maintainable E2E test suite for your web application using
Playwright and POM.
To customize this framework according to your needs,feel free to fork the Github repository at GitHub Repository and modify the code as per your convenience.
No comments:
Post a Comment
Note: Only a member of this blog may post a comment.